Can C Functions Be Overloaded Based on Their Return Value?
Dec 01, 2024 am 01:49 AMOverloading Functions Based on Return Value in C
Overloading functions based on parameters is a common practice in C . However, overloading based on the return value is also possible, allowing you to create functions that behave differently depending on how the return value is used.
There are several methods to achieve this:
Explicit Typing of Calls
Use different types for the literals passed to the function. For example, for a function returning an integer or a string depending on usage:
int mul(int, int); std::string mul(char, int); int n = mul(6, 3); // Function called with int return value std::string s = mul('6', 3); // Function called with string return value
Dummy Pointer Approach
Add a dummy pointer parameter to each function, forcing the compiler to choose the correct version based on the return value type:
int mul(int*, int, int); std::string mul(std::string*, char, int); int n = mul((int*)NULL, 6, 3); // Function called with int return value std::string s = mul((std::string*)NULL, '6', 3); // Function called with string return value
Template Specialization for Return Value
Create template functions and specialize them for specific return types:
template<typename T> T mul(int, int) { // Generic function with a dummy member variable that will cause a compilation error // if not specialized const int k = 25; k = 36; } template<> int mul<int>(int, int) { return i * j; } template<> std::string mul<std::string>(int, int) { return std::string(j, static_cast<char>(i)); } int n = mul<int>(6, 3); // Function called with int return value std::string s = mul<std::string>('6', 3); // Function called with string return value
This method requires explicitly specifying the return type when calling the function to avoid ambiguities.
Template Specialization with Multiple Parameters
To overload based on different parameters for the same return value type, create separate templates for each parameter combination:
template<typename T> T mul(int, int) { // Generic function with a dummy member variable that will cause a compilation error // if not specialized const int k = 25; k = 36; } template<> int mul<int>(int, int) { return i * j; } template<typename T> T mul(char, int) { // Generic function with a dummy member variable that will cause a compilation error // if not specialized const int k = 25; k = 36; } template<> std::string mul<std::string>(char, int) { return std::string(j, static_cast<char>(i)); } int n = mul<int>(6, 3); // n = 18 std::string s = mul<std::string>('6', 3); // s = "666"
Using these techniques, you can effectively overload functions based on their return value, allowing for more versatile and flexible code.
The above is the detailed content of Can C Functions Be Overloaded Based on Their Return Value?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
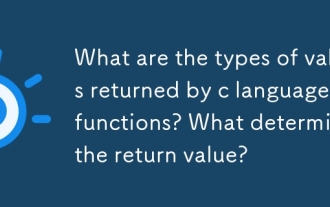
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
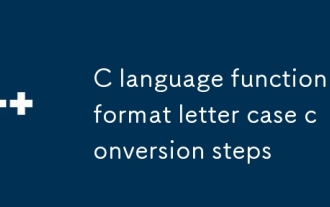
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
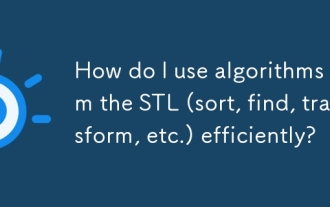
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
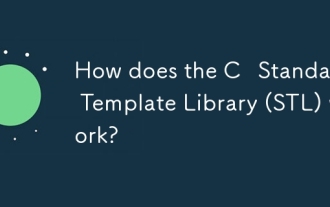
How does the C Standard Template Library (STL) work?
