How Can I Convert a Struct Pointer to an interface{} Value in Go?
Converting Struct Pointers to Interface{} Values
Given the following code snippet:
type foo struct {} func bar(baz interface{}) {}
where both foo and bar are immutable by design, how can you convert a &foo{} struct pointer into an interface{} value and subsequently utilize it as a parameter for the bar function?
Solution
Casting a struct pointer to an interface{} value is straightforward:
f := &foo{} bar(f) // Every type implements interface{}, so no special action is required.
Recovering the original *foo pointer from the interface{} value requires either type assertion or a type switch.
Type Assertion:
func bar(baz interface{}) { f, ok := baz.(*foo) if !ok { // The assertion failed because baz was not of type *foo. } // f is of type *foo. }
Type Switch:
func bar(baz interface{}) { switch f := baz.(type) { case *foo: // f is of type *foo. default: // f is some other type. } }
The above is the detailed content of How Can I Convert a Struct Pointer to an interface{} Value in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
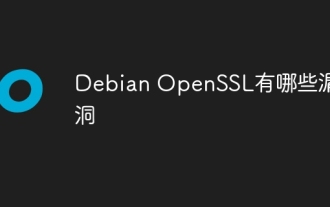
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
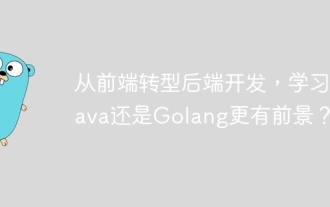
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
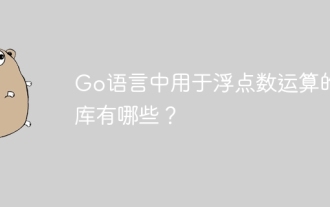
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
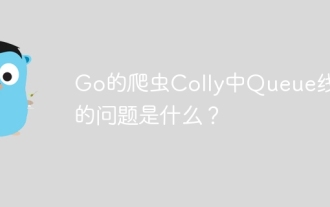
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
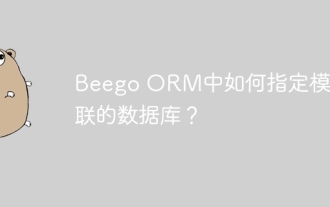
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
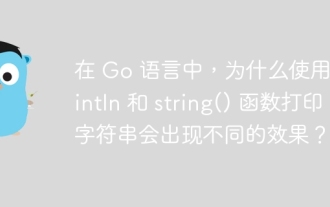
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
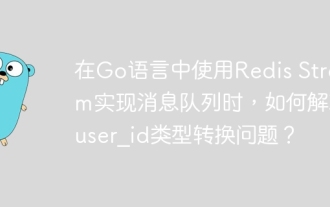
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
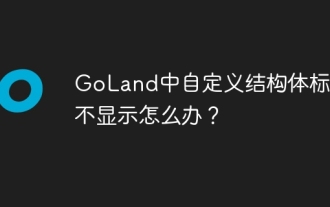
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
