Securing PHP Web Applications: Hands-On Practices
Introduction
Learn the essential security practices every PHP developer must adopt to safeguard web applications. From sanitizing inputs to implementing HTTPS and using modern security headers, this guide provides practical examples and step-by-step instructions to mitigate vulnerabilities like SQL injection, XSS, and CSRF.
Table of Contents
- Input Validation and Sanitization
- Using Prepared Statements for SQL Queries
- Secure Password Storage
- Preventing XSS Attacks
- Implementing CSRF Protection
- Setting HTTP Security Headers
- Managing Secure PHP Sessions
- Configuring Error Reporting Safely
- Enforcing HTTPS with SSL/TLS
- Keeping PHP and Libraries Updated
1. Input Validation and Sanitization
Never trust user inputs; validate and sanitize them before processing.
Example: Validating and sanitizing a contact form input
<?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = filter_input(INPUT_POST, 'name', FILTER_SANITIZE_STRING); $email = filter_input(INPUT_POST, 'email', FILTER_SANITIZE_EMAIL); if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { echo "Invalid email address!"; } else { echo "Name: " . htmlspecialchars($name) . "<br>Email: " . htmlspecialchars($email); } } ?>
Explanation:
- filter_input() sanitizes the input by removing harmful characters.
- FILTER_VALIDATE_EMAIL checks if the input is a valid email.
- htmlspecialchars() prevents HTML injection by escaping special characters.
2. Use Prepared Statements for Database Queries
Protect against SQL Injection attacks.
Example: Using PDO with prepared statements
<?php try { $pdo = new PDO('mysql:host=localhost;dbname=testdb', 'root', ''); $pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); $stmt = $pdo->prepare("SELECT * FROM users WHERE email = :email"); $stmt->bindParam(':email', $email, PDO::PARAM_STR); $email = $_POST['email']; $stmt->execute(); $user = $stmt->fetch(PDO::FETCH_ASSOC); if ($user) { echo "Welcome, " . htmlspecialchars($user['name']); } else { echo "User not found."; } } catch (PDOException $e) { echo "Database error: " . $e->getMessage(); } ?>
Explanation:
- Prepared statements ensure query parameters are escaped properly, preventing SQL injection.
- bindParam() securely binds the variable to the query.
Conclusion
By following these security best practices, you can build robust PHP applications that protect both user data and server integrity. Security isn't a one-time task but an ongoing process requiring regular updates, audits, and adherence to coding standards. Adopt these methods to enhance the trustworthiness and reliability of your applications.
If you'd like to explore best practices more, Click Here.
Stay Connected!
- Connect with me on LinkedIn to discuss ideas or projects.
- Check out my Portfolio for exciting projects.
- Give my GitHub repositories a star ⭐ on GitHub if you find them useful!
Your support and feedback mean a lot! ?
The above is the detailed content of Securing PHP Web Applications: Hands-On Practices. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










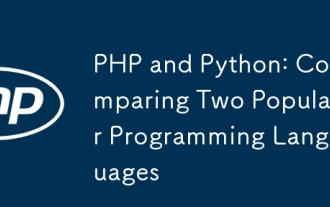
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
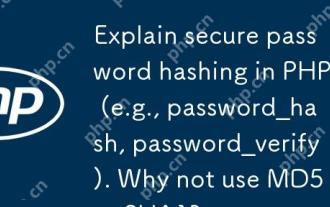
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
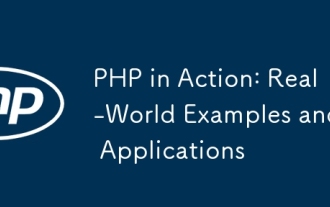
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
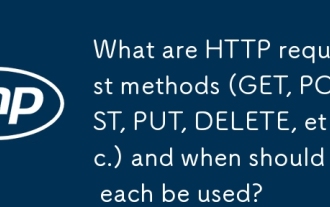
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
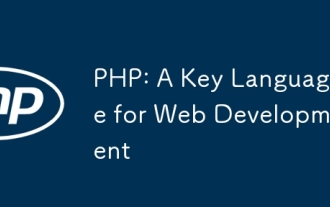
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
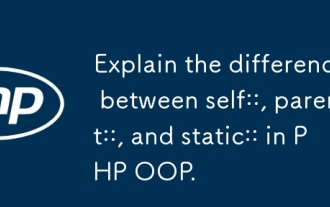
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
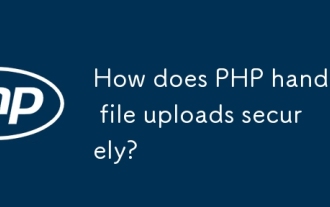
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
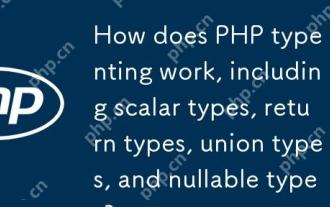
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
