How to Unmarshal JSON with Variable Array Field Types in Go?
Unmarshalling JSON with a Variable Array Field Type in Go
In Go, JSON data is unmarshalled into a predefined struct. However, certain API responses can present challenges when the type of a key's value can vary between requests. For instance, an API might return either inline objects or object references for a specific key.
To handle this variability, consider using the following approach:
type Data struct { MyKey []interface{} `json:"mykey"` }
This structure unmarshals both inline objects and object references into an array of interfaces. By using a type switch after unmarshalling, you can determine the actual type of each element in the array:
for _, v := range data.MyKey { switch x := v.(type) { case string: fmt.Println("Got a string: ", x) case map[string]interface{}: fmt.Printf("Got an object: %#v\n", x) } }
This method allows you to parse JSON data with variable array field types in a flexible and idiomatic manner.
The above is the detailed content of How to Unmarshal JSON with Variable Array Field Types in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
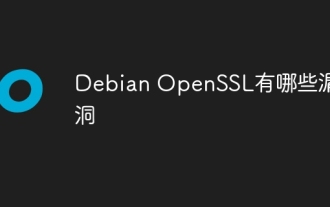
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
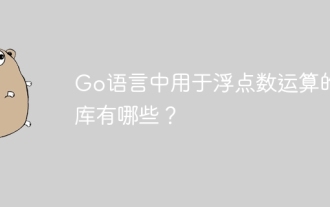
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
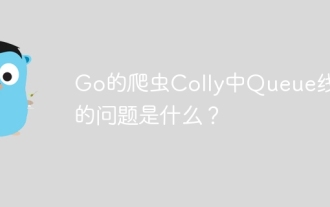
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
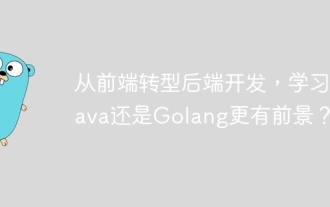
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
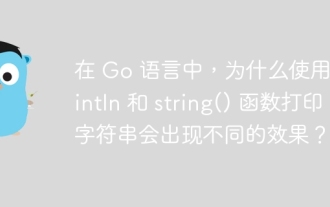
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
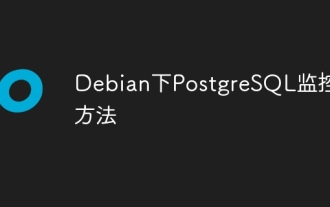
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
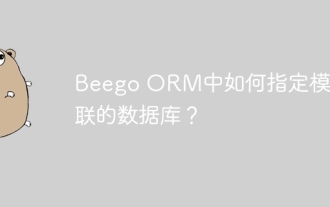
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
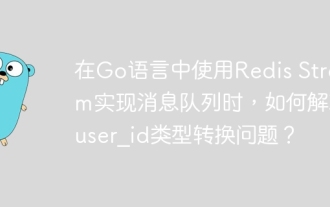
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
