


How Can I Avoid Closure Issues When Assigning Click Handlers in JavaScript Loops?
Addressing Closure Issues in Click Handler Assignment within Loops
When assigning click handlers to multiple elements in a loop, a common pitfall arises due to closures in JavaScript. The code provided exhibits this issue:
$(document).ready(function(){ for(var i = 0; i < 20; i++) { $('#question' + i).click( function(){ alert('you clicked ' + i); }); } });
This code aims to display the clicked element's index when a click occurs. However, it incorrectly shows 'you clicked 20' for every click, instead of displaying the actual index. This behavior stems from the closure created in the loop.
Solution: Employing Callback Functions
To resolve this issue, we can utilize callback functions as shown below:
function createCallback( i ){ return function(){ alert('you clicked' + i); } } $(document).ready(function(){ for(var i = 0; i < 20; i++) { $('#question' + i).click( createCallback( i ) ); } });
In this updated version, a callback function is created within the loop. This function captures the current value of 'i', ensuring that the correct index is displayed when the element is clicked.
Leveraging ES6's let Keyword
If ES6 syntax is available, we can utilize the let keyword to achieve the same result more concisely:
for(let i = 0; i < 20; i++) { $('#question' + i).click( function(){ alert('you clicked ' + i); }); }
The let keyword ensures that 'i' is scoped locally within the loop, avoiding closure issues and displaying the correct clicked index upon each element's activation.
The above is the detailed content of How Can I Avoid Closure Issues When Assigning Click Handlers in JavaScript Loops?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
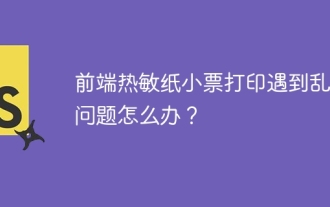
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
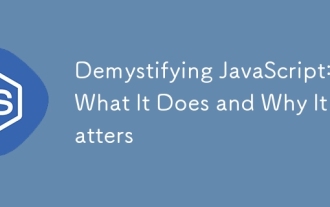
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
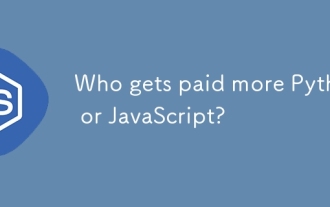
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
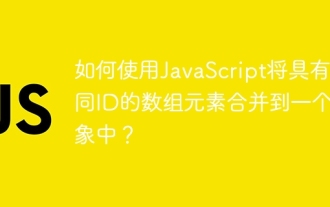
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
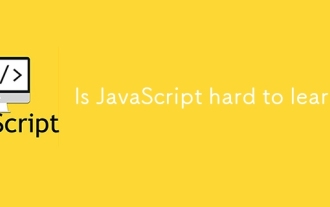
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
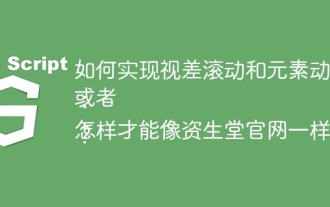
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
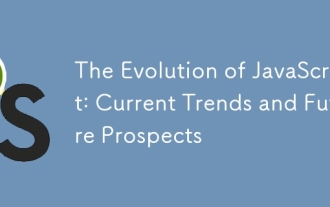
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
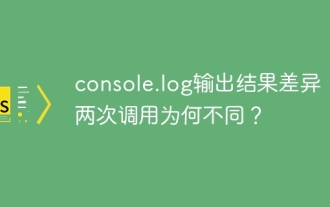
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
