Can You Loop in Python Without an Iterator Variable?
Looping Without an Iterator Variable
In Python, you can iterate through a sequence using a for loop, which typically involves using an iterator variable. However, some scenarios may arise where you want to loop a fixed number of times without the need for an iterator.
Consider the following code snippet:
1 2 |
|
In this example, we iterate through the range from 0 to 4 and print "Hello" five times. However, you may wonder if it's possible to accomplish this without using the i variable.
Direct Answer
There is no direct way in Python to loop without an iterator variable. The range() function requires an iterator variable to specify the loop boundaries.
Workarounds
While there is no native Python solution, you can employ workarounds to simulate looping without an iterator.
Using a Lambda Function:
1 2 3 4 5 |
|
This approach involves creating a nested function that takes in the number of iterations and a callback function. The loop function then executes the callback function n times.
Using the Underscore Variable (_):
You can use the _ variable, which is a special variable that represents the last returned value. However, be aware that using _ may not be ideal as it can potentially cause confusion and interfere with variable assignments.
1 2 |
|
Conclusion
Although there is no direct way to loop without an iterator in Python, these workarounds provide alternative approaches to simulate similar behavior. Ultimately, the choice of method depends on the specific requirements and preferences of your application.
The above is the detailed content of Can You Loop in Python Without an Iterator Variable?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










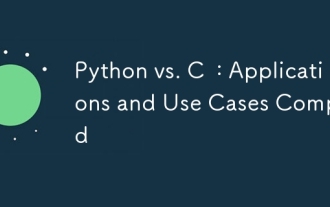
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
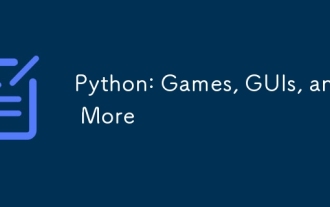
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
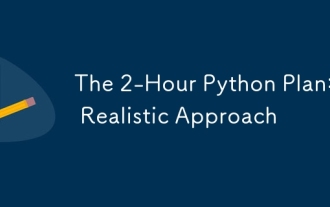
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
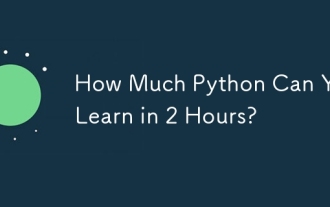
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
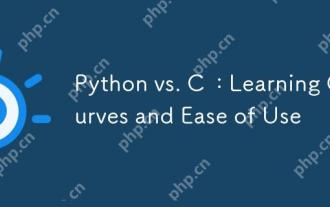
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
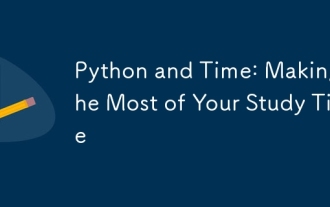
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
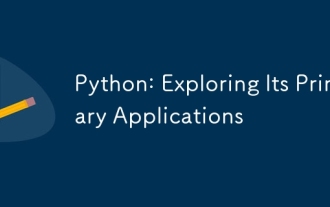
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
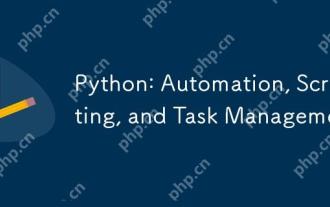
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
