


How Can I Efficiently Check if All Elements in a Python List are Equal?
Checking Equality of List Elements
In Python, determining if all elements within a list are equal can be achieved using various approaches. One recommended method involves utilizing the itertools.groupby() function. This function groups consecutive elements with identical values, returning a sequence of tuples containing group keys (element values) and their respective groups.
from itertools import groupby def all_equal(iterable): g = groupby(iterable) return next(g, True) and not next(g, False)
This code checks whether there's only one group within the list, indicating that all elements are equal. If that's the case, it returns True; otherwise, it returns False using the short-circuit evaluation property.
Alternately, you can use a more concise solution without groupby():
def all_equal(iterator): iterator = iter(iterator) try: first = next(iterator) except StopIteration: return True return all(first == x for x in iterator)
This approach initializes an iterator and retrieves the first element. Subsequently, it iterates over the remaining elements, comparing them to the first. If any difference is encountered, the function returns False; otherwise, it returns True.
While these methods are efficient, there are alternative one-liners you can consider:
- Convert to a Set: Check if the length of the resultant set is less than or equal to 1.
- Compare to the First Item: Compare the list to a list containing only the first item repeated.
- Count Occurrences of First Item: Check if the count of the first item in the list is equal to the list's length.
These alternatives may be less memory-intensive and yield different performance characteristics. However, it's important to consider their limitations, such as potential memory copies and varying efficiency depending on the list's nature.
The above is the detailed content of How Can I Efficiently Check if All Elements in a Python List are Equal?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










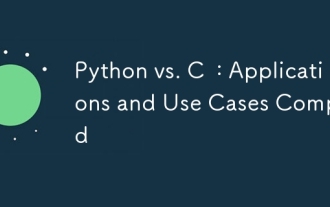
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
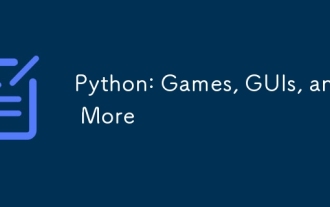
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
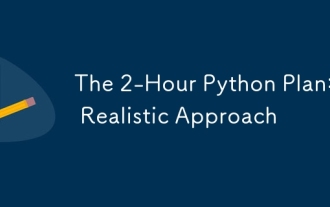
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
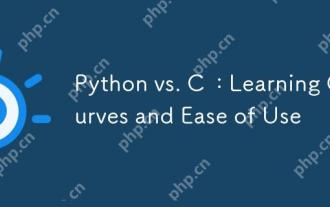
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
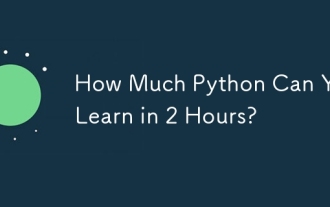
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
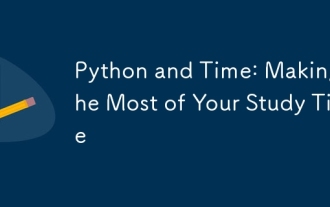
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
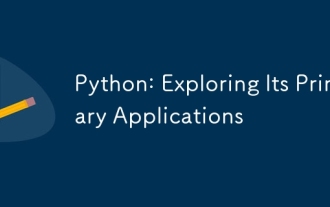
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
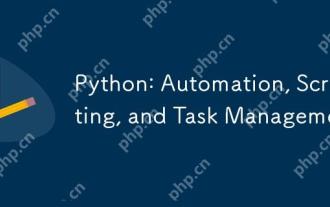
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
