How to Ensure Complete Data Transmission in a TCP Proxy?
How to Resolve Uncertainty in TCP Proxy Data Transmission
When designing a TCP proxy that forwards requests and responses between a client and a server, it is crucial to ensure that data is transmitted correctly. A key challenge arises when determining whether all the necessary information has been received from the server to forward to the client.
One common approach is to assume that when a read operation from the server connection returns zero, it signifies the end of data transmission. However, if the server is writing data to the socket one byte at a time, the proxy may mistakenly conclude it has received all the data. This is because the read operation may occur rapidly, reading only partial data before the server has finished transmitting.
Addressing the Issue
To resolve this issue, a more robust approach is to use buffering and a timer. By reading data into a buffer and checking its length periodically, the proxy can determine when a complete response has been received. The timer ensures that the proxy does not wait indefinitely in case the server has stalled or disconnected.
An additional consideration is the potential for a network partition. If the server becomes temporarily unavailable, the proxy should not assume it has received all the data. Instead, it should handle the timeout exception appropriately to maintain connection stability.
Code Optimization
The code provided in the question can be optimized by using a separate goroutine for each direction of data transfer. This allows the proxy to handle data in parallel for both the client and server connections.
Example Implementation
The following code snippet demonstrates an optimized proxy implementation using goroutines:
package main import ( "fmt" "net" ) type Proxy struct { ServerConn *net.TCPConn ClientConn *net.TCPConn } func (p *Proxy) Proxy() { fmt.Println("Running proxy...") go func() { _, err := io.Copy(p.ServerConn, p.ClientConn) if err != nil { fmt.Println(err) } }() go func() { _, err := io.Copy(p.ClientConn, p.ServerConn) if err != nil { fmt.Println(err) } }() } func main() { // Initialize the TCP connections serverConn, clientConn, err := net.DialTCP("tcp", nil, &net.TCPAddr{IP: net.ParseIP("127.0.0.1"), Port: 8080}) if err != nil { fmt.Println(err) return } proxy := Proxy{serverConn, clientConn} proxy.Proxy() }
This code is more efficient and handles errors more gracefully than the original provided code.
The above is the detailed content of How to Ensure Complete Data Transmission in a TCP Proxy?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










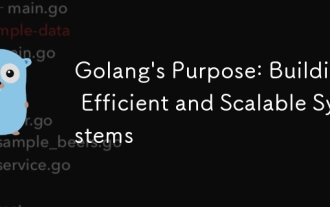
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
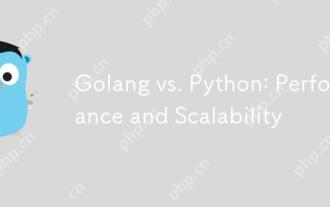
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
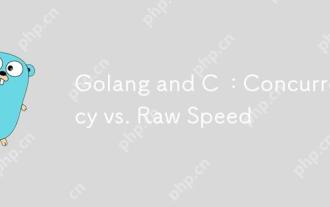
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
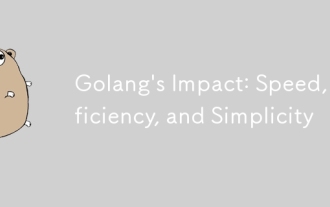
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
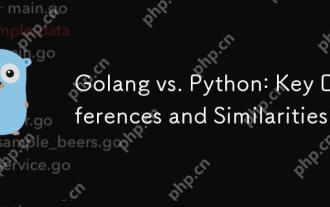
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
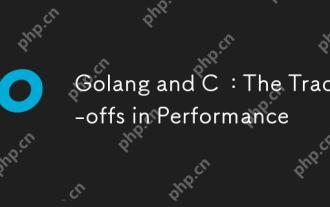
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
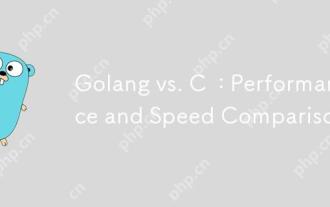
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
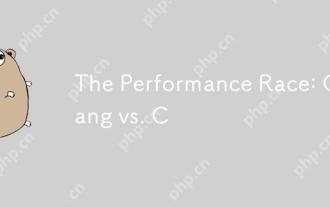
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
