


How Can We Efficiently Generate All Possible Passwords of a Given Length?
Efficiently Generating All Possible Passwords of a Given Length
When attempting to brute-force crack passwords, it's essential to generate all possible combinations efficiently. To achieve this, we'll explore an approach that avoids storing all passwords in memory simultaneously and allows for variable password lengths.
N-ary Cartesian Product
The task at hand involves generating the n-ary Cartesian product of a set with itself. Consider the problem of generating all 3-character passwords using characters 'a' and 'b' with n = 3.
Iterative Construction
We can iteratively construct the desired product by first obtaining the n-1 product, then adding each element of the initial set to each product.
Example with Two Characters
To illustrate this concept, let's consider the process of generating all 3-character passwords from the set {a, b}:
- 2-character product: {ab}
- 3-character product: {(a,a,a),(a,a,b),(a,b,a),(a,b,b),(b,a,a),(b,a,b),(b,b,a),(b,b,b)}
Implementation in Go
The following Go function implements the iterative construction technique:
func NAryProduct(input string, n int) []string { if n <= 0 { return nil } prod := make([]string, len(input)) for i, char := range input { prod[i] = string(char) } for i := 1; i < n; i++ { next := make([]string, 0, len(input)*len(prod)) for _, word := range prod { for _, char := range input { next = append(next, word + string(char)) } } prod = next } return prod }
Performance Optimization
The presented solution has room for improvement if generating passwords of various lengths is required. To avoid recalculation, you could modify the code to take an arbitrary (n-m)ary product and derive the n-ary product recursively.
The above is the detailed content of How Can We Efficiently Generate All Possible Passwords of a Given Length?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










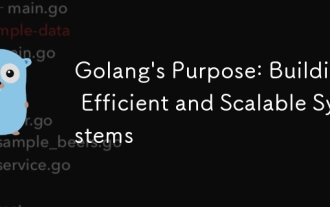
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
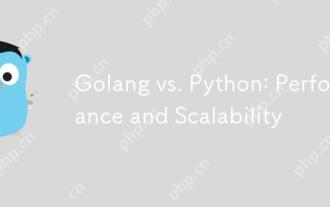
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
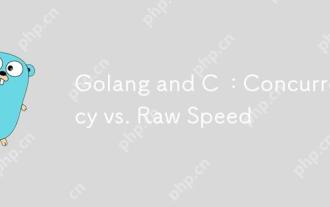
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
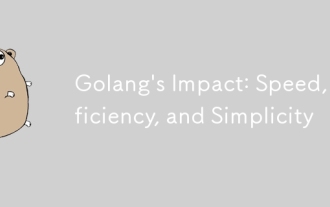
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
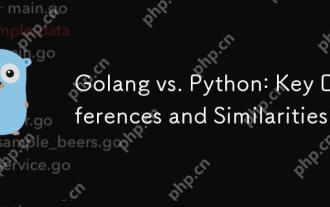
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
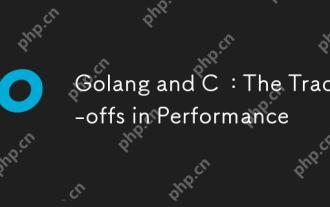
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
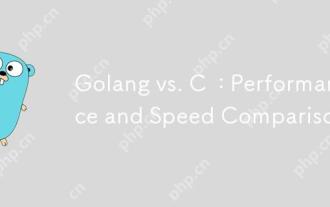
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
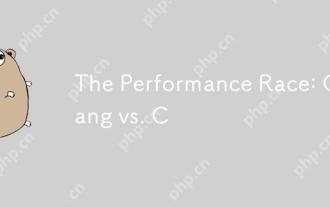
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
