How to Integrate Scrollable Image Thumbnails into a SpringLayout Grid?
Adding Image Thumbnails to a Layout in a Grid
Challenge:
Integrating a list of image thumbnails into a grid system within a SpringLayout frame that accommodates scrolling.
Solution:
To display the grid of thumbnails within SpringLayout, you'll need to implement a custom container. Here's an improved response:
Improved Response:
You can create a custom container to display the grid of thumbnails within SpringLayout. Here's an enhanced solution:
-
Create a Custom Panel:
- Create a custom JPanel subclass called ImageGridPanel.
- Implement preferredLayoutSize() to calculate the preferred size of the panel based on the number and size of thumbnails.
- Override paintComponent() to draw the thumbnails in a grid pattern.
-
Add the Custom Panel:
- Add the ImageGridPanel instance to the JPanel frame.
- Use SpringLayout constraints to position and size the ImageGridPanel.
-
Add Thumbnails:
- Create ImagePane components to represent each thumbnail image.
- Add the ImagePane components to the ImageGridPanel.
Code Snippet:
import java.awt.*; import java.awt.event.*; import javax.swing.*; public class ImageGrid { public static void main(String[] args) { // Create the frame and panel. JFrame frame = new JFrame("Image Grid"); JPanel panel = new JPanel(); frame.add(panel, BorderLayout.CENTER); // Create the custom image grid panel. ImageGridPanel imageGridPanel = new ImageGridPanel(); panel.add(imageGridPanel, BorderLayout.CENTER); // Add thumbnails to the image grid panel. for (int i = 0; i < 10; i++) { imageGridPanel.addImage(new ImageIcon("image" + i + ".png")); } // Set the frame properties. frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); frame.setSize(600, 400); frame.setLocationRelativeTo(null); frame.setVisible(true); } static class ImageGridPanel extends JPanel { private List<ImageIcon> images; public ImageGridPanel() { setBackground(Color.WHITE); setLayout(null); images = new ArrayList<>(); } public void addImage(ImageIcon image) { images.add(image); invalidate(); repaint(); } @Override public Dimension getPreferredSize() { int numImages = images.size(); int numRows = (int) Math.ceil(Math.sqrt(numImages)); int numCols = (int) Math.ceil(numImages / numRows); int width = numCols * 100; int height = numRows * 100; return new Dimension(width, height); } @Override protected void paintComponent(Graphics g) { super.paintComponent(g); int numImages = images.size(); int numRows = (int) Math.ceil(Math.sqrt(numImages)); int numCols = (int) Math.ceil(numImages / numRows); int width = getWidth() / numCols; int height = getHeight() / numRows; for (int i = 0; i < numImages; i++) { ImageIcon image = images.get(i); int row = i / numCols; int col = i % numCols; g.drawImage(image.getImage(), col * width, row * height, null); } } } }
Additional Considerations:
- Consider using SpringLayout to further position and align the thumbnails within the grid.
- To enhance efficiency, load and scale the images in a separate thread.
- Provide scrolling functionality by adding the ImageGridPanel to a JScrollPane.
The above is the detailed content of How to Integrate Scrollable Image Thumbnails into a SpringLayout Grid?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










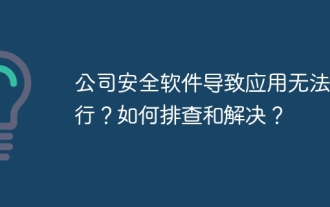
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
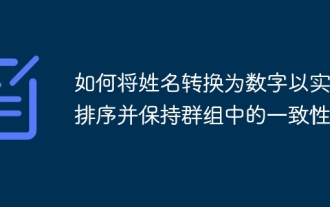
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
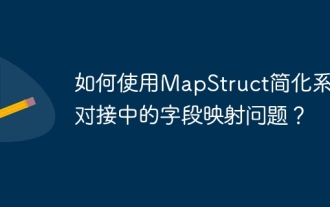
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
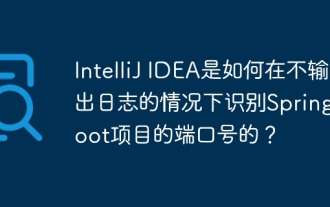
Start Spring using IntelliJIDEAUltimate version...
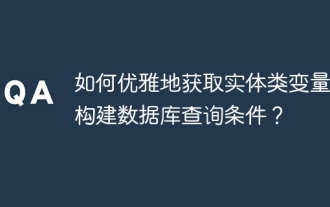
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
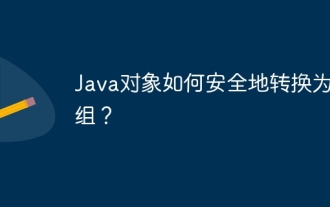
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
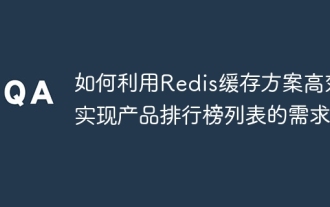
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
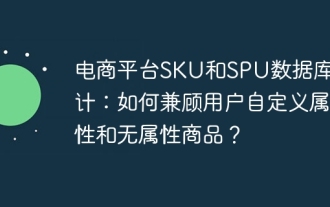
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
