


Understanding JWT Authentication: Spring Securitys Architecture and Go Implementation
After setting up JWT stateless authentication (available here), I wanted to understand what happens under Spring Security's abstractions by identifying key components and their interactions. To make this exploration more engaging, I reimplemented a minimal version in Go using the standard HTTP library. By breaking down three core flows - registration, token generation, and protected resource access - and rebuilding them in Go, I set out to map Spring Security's authentication patterns to simpler components.
This post focuses specifically on authentication flows - how the system verifies user identity - rather than authorization. We'll explore the flows with sequence diagrams that trace requests through different components in Spring Security's architecture.
Main Components
The system provides three endpoints:
- User Registration: Accepts username and password from new users
- Token Generation (Login): Creates a JWT token when users successfully log in with valid credentials
- Protected Access: Enables authenticated users to access protected resources using their token. The getAuthenticatedUser endpoint serves as an example, returning profile information for the authenticated token holder
In the following sections, I explain the core components involved in each flow, with a sequence diagram for each.
Registration Flow
A registration request containing username and password passes through the Spring Security filter chain, where minimal processing occurs since the registration endpoint was configured to not require authentication in SecurityConfiguration. The request then moves through Spring's DispatcherServlet, which routes it to the appropriate method in UserController based on the URL pattern. The request reaches UserController's register endpoint, where the user information is stored along with a hashed password.
Token Generation Flow
A login request containing username and password passes through the Spring Security filter chain, where minimal processing occurs as this endpoint is also configured to not require authentication in SecurityConfiguration. The request moves through Spring's DispatcherServlet to UserController's login endpoint, which delegates to AuthenticationManager. Using the configured beans defined in ApplicationConfiguration, AuthenticationManager verifies the provided credentials against stored ones. After successful authentication, the UserController uses JwtService to generate a JWT token containing the user's information and metadata like creation time, which is returned to the client for subsequent authenticated requests.
Protected Resource Access Flow
Successful Authentication Flow (200)
Failed Authentication Flow (401)
When a request containing a JWT token in its Authorization header arrives, it passes through the JwtAuthenticationFilter - a custom defined OncePerRequestFilter - which processes the token using JwtService. If valid, the filter retrieves the user via UserDetailsService configured in ApplicationConfiguration and sets the authentication in SecurityContextHolder. If the token is missing or invalid, the filter allows the request to continue without setting authentication.
Later in the chain, AuthorizationFilter checks if the request is properly authenticated via SecurityContextHolder. When it detects missing authentication, it throws an AccessDeniedException. This exception is caught by ExceptionTranslationFilter, which checks if the user is anonymous and delegates to the configured JwtAuthenticationEntryPoint in SecurityConfiguration to return a 401 Unauthorized response.
If all filters pass, the request reaches Spring's DispatcherServlet which routes it to the getAuthenticatedUser endpoint in UserController. This endpoint retrieves the authenticated user information from SecurityContextHolder that was populated during the filter chain process.
Note: Spring Security employs a rich ecosystem of filters and specialized components to handle various security concerns. To understand the core authentication flow, I only focused on the key players in JWT token validation and user authentication.
Go Implementation: Mapping Components
The Go implementation provides similar functionality through a simplified architecture that maps to key Spring Security components:
FilterChain
- Provides a minimal version of Spring Security's filter chain
- Processes filters sequentially for each request
- Uses a per-request chain instance (VirtualFilterChain) for thread safety
Dispatcher
- Maps to Spring's DispatcherServlet
- Routes requests to appropriate handlers after security filter processing
Authentication Context
- Uses Go's context package to store authentication state per request
- Maps to Spring's SecurityContextHolder
JwtFilter
- Direct equivalent to Spring's JwtAuthenticationFilter
- Extracts and validates JWT tokens
- Populates authentication context on successful validation
AuthenticationFilter
- Simplified version of Spring's AuthorizationFilter
- Solely focusing on authentication verification
- Checks authentication context and returns 401 if missing
JwtService
- Similar to Spring's JwtService
- Handles token generation and validation
- Uses same core JWT operations but with simpler configuration
Test Coverage
Both implementations include integration tests (auth_test.go and AuthTest.java) verifying key authentication scenarios:
Registration Flow
- Successful user registration with valid credentials
- Duplicate username registration attempt
Login Flow
- Successful login with valid credentials
- Login attempt with non-existent username
- Login attempt with incorrect password
Protected Resource Access
- Successful access with valid token
- Access attempt without auth header
- Access attempt with invalid token format
- Access attempt with expired token
- Access attempt with valid token format but non-existent user
The Java implementation includes detailed comments explaining the flow of each test scenario through Spring Security's filter chain. These same flows are replicated in the Go implementation using equivalent components.
Journey Summary
I looked at Spring Security's JWT auth by breaking it down into flows and test cases. Then I mapped these patterns to Go components. Integration tests showed me how requests flow through Spring Security's filter chain and components. Building simple versions of these patterns helped me understand Spring Security's design. The tests proved both implementations handle authentication the same way. Through analyzing, testing, and rebuilding, I gained a deeper understanding of how Spring Security's authentication works.
The above is the detailed content of Understanding JWT Authentication: Spring Securitys Architecture and Go Implementation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










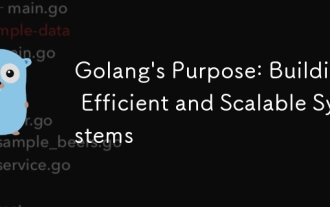
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
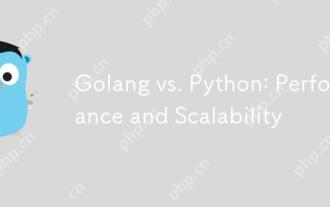
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
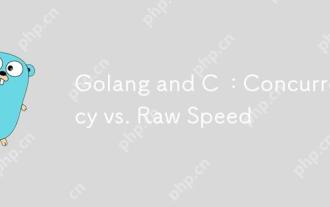
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
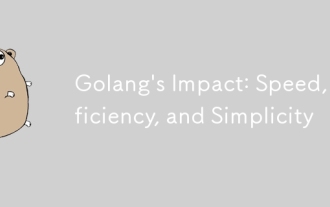
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
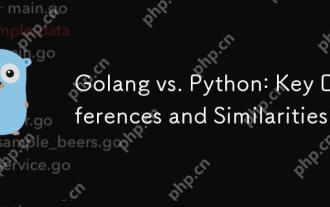
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
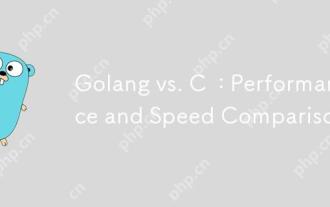
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
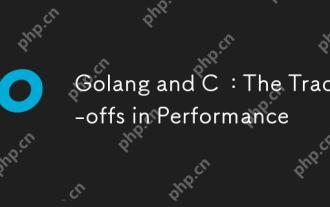
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
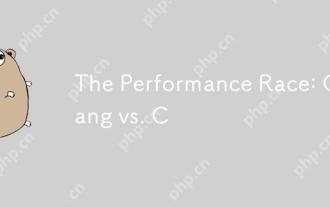
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
