How Can I Easily Convert Comma-Separated Strings to Lists in Java?
Effortlessly Convert Comma-Separated Strings to Lists
Java offers a convenient method to transform comma-delimited strings into List objects. This eliminates the need for custom code and simplifies the process.
Suppose we have a string like "item1 , item2 , item3". To convert it into a List of strings, we can use the following code snippet:
String commaSeparated = "item1 , item2 , item3"; List<String> items = Arrays.asList(str.split("\s*,\s*"));
The heart of this code is the split() method. It divides the string into individual substrings using the specified delimiter. In our case, the delimiter is a comma with optional whitespace on both sides.
The resulting substrings are then stored in an array, which is then wrapped into a List object. This List provides convenient access to the individual elements, making it easier to work with the data.
Note that the resulting List is initially just a wrapper around an array. If you need a true ArrayList with full modification capabilities, you can use the following code:
List<String> items = new ArrayList<>(Arrays.asList(str.split("\s*,\s*")));
Converting comma-separated strings to Lists in Java is a simple and efficient operation. By leveraging the built-in split() method, developers can save time and effort while ensuring accuracy in data manipulation.
The above is the detailed content of How Can I Easily Convert Comma-Separated Strings to Lists in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










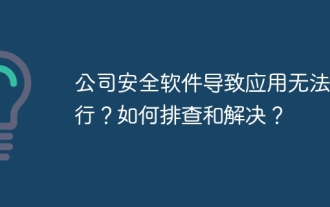
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
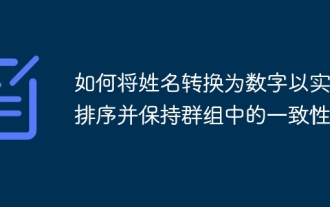
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
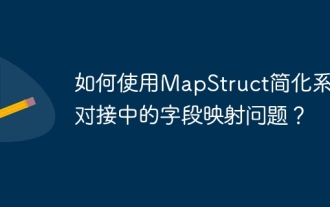
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
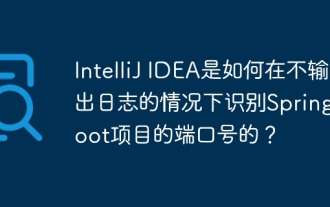
Start Spring using IntelliJIDEAUltimate version...
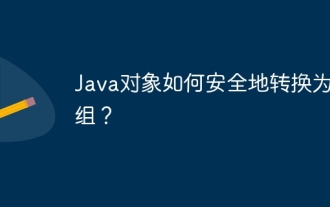
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
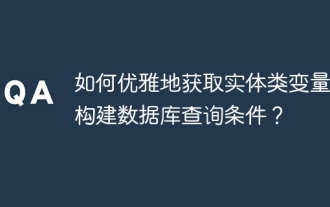
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
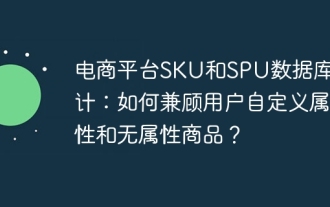
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
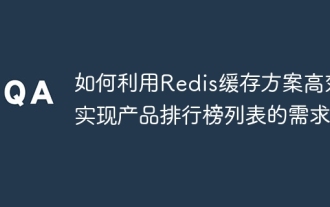
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
