


How to Handle JSON Data and Images in Multipart/Form-Data Requests with Gin?
Go: Handling JSON Data and Image in a Form with Gin
Gin is a popular web framework in Go that simplifies handling HTTP requests. This article addresses a specific issue with parsing both JSON data and an image from a multipart/form-data request using Gin's binding mechanisms.
Request Handling Code
The request handler function in Gin receives and handles HTTP requests. In this case, it expects to receive a multipart request containing both JSON data and an image file.
func (h *Handlers) UpdateProfile() gin.HandlerFunc { type request struct { Username string `json:"username" binding:"required,min=4,max=20"` Description string `json:"description" binding:"required,max=100"` } return func(c *gin.Context) { var updateRequest request // Bind JSON data to `updateRequest` struct. if err := c.BindJSON(&updateRequest); err != nil { // Handle error here... return } // Get the image file from the request. avatar, err := c.FormFile("avatar") if err != nil { // Handle error here... return } // Validate file size and content type. if avatar.Size > 3<<20 || !avatar.Header.Get("Content-Type") { // if avatar size more than 3mb // Handle error here... return } // Handle image processing and database operations here... // Save username, description, and image to a database. c.IndentedJSON(http.StatusNoContent, gin.H{"message": "successful update"}) } }
Test Case
A unit test is included to verify the handler's functionality. It sets up a mock request with a multipart/form-data body. The request contains JSON data and an image.
func TestUser_UpdateProfile(t *testing.T) { type testCase struct { name string image io.Reader username string description string expectedStatusCode int } // Set up mock request with multipart/form-data body. // ... for _, tc := range testCases { // ... w := httptest.NewRecorder() router.ServeHTTP(w, req) assert.Equal(t, tc.expectedStatusCode, w.Result().StatusCode) } }
Error During Test
During the test, an error occurred due to an invalid character in the request body. The error message was "Error #01: invalid character '-' in numeric literal."
Root Cause
Gin's c.BindJSON function is used to parse JSON data from the request body. However, it assumes that the request body starts with valid JSON. In the case of a multipart/form-data request, the body starts with a boundary (--30b24345de...), which is an invalid character for a JSON literal.
Solution
To resolve this issue, we can use Gin's c.ShouldBind function with binding.FormMultipart to explicitly bind to the multipart/form-data body. This allows Gin to properly parse both the JSON data and the image file.
// Bind JSON data and image file to `updateRequest` struct. if err := c.ShouldBindWith(&updateRequest, binding.FormMultipart); err != nil { // Handle error here... return }
Conclusion
This article demonstrates how to handle JSON data and an image file simultaneously in a multipart/form-data request with Gin. It highlights the importance of using the correct binding method (c.ShouldBindWith(..., binding.FormMultipart)) to avoid parsing errors.
The above is the detailed content of How to Handle JSON Data and Images in Multipart/Form-Data Requests with Gin?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
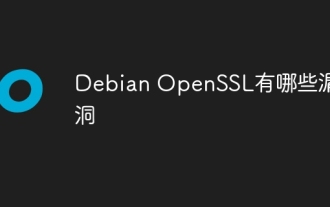
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
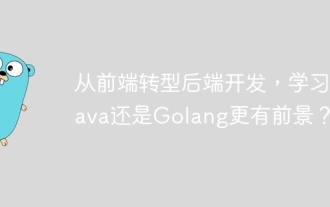
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
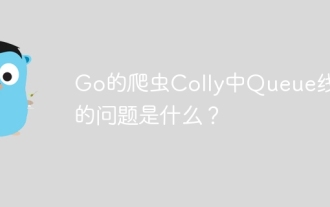
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
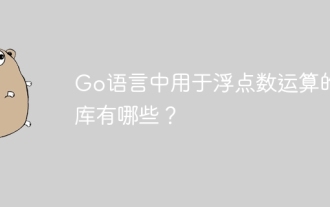
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
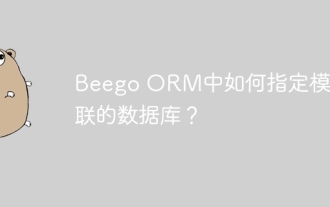
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
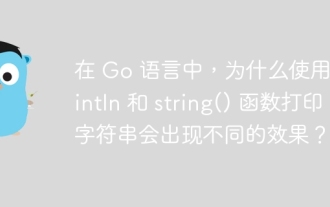
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
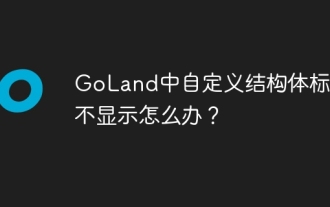
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
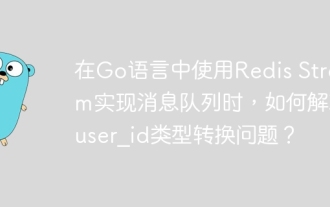
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
