How Can I Safely Convert an interface{} to a Map in Go?
Converting Interface{} to a Map using Go
In Go, a common task is to convert an interface{} to a specific type, such as a map. This can be challenging if you're unsure of the underlying type stored in the interface.
Consider this scenario where you want to create a function that can handle different input types: structs, slices of structs, and maps where the keys are strings and the values are structs.
Using reflection, you can check the type of the interface{} value. If it's a slice, you can iterate over the elements and process each struct. However, you encounter an error when trying to handle a map.
func process(in interface{}, isSlice bool, isMap bool) { v := reflect.ValueOf(in) if isSlice { for i := 0; i < v.Len(); i++ { strct := v.Index(i).Interface() // ... process struct } return } if isMap { fmt.Printf("Type: %v\n", v) // map[] for _, s := range v { // Error: cannot range over v (type reflect.Value) fmt.Printf("Value: %v\n", s.Interface()) } } }
The error occurs because v is a reflect.Value, not the actual map you want to iterate over. To work around this, you can use type assertion or, if you prefer reflection, use the Value.MapKeys method.
Using Type Assertion:
v, ok := in.(map[string]*Book) if !ok { // Handle error } for _, s := range v { fmt.Printf("Value: %v\n", s) }
Using Reflection (Value.MapKeys):
keys := v.MapKeys() for _, key := range keys { value := v.MapIndex(key).Interface() fmt.Printf("Key: %v, Value: %v\n", key, value) }
By using type assertion or Value.MapKeys, you can convert an interface{} to a map and iterate over its elements without encountering errors. Remember, while reflection provides a powerful way to inspect and manipulate types dynamically, it's often better to use type switching for common type conversion scenarios.
The above is the detailed content of How Can I Safely Convert an interface{} to a Map in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










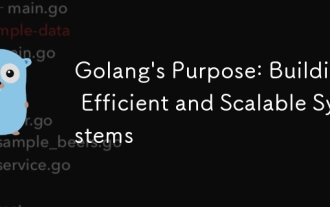
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
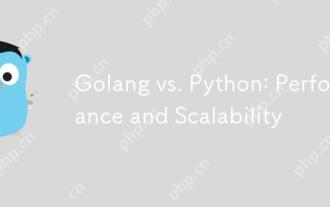
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
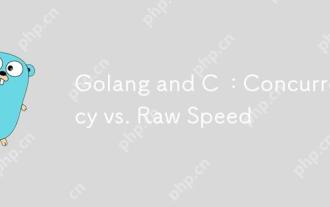
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
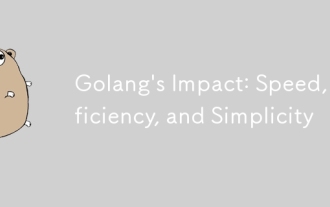
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
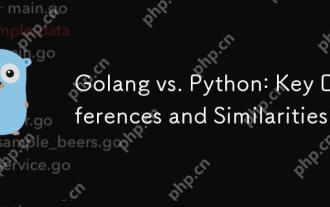
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
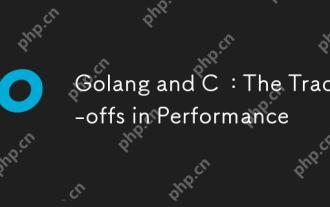
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
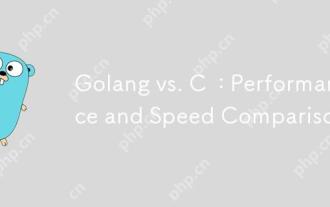
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
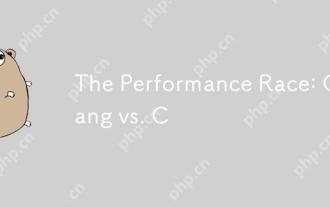
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
