


How Can I Compare `interface{}` Values for Equality in Go, Especially with Custom Structs?
Equality Checking for Interface{}
In Go, comparing interface{} values can be a bit tricky, especially when dealing with custom structs. This article aims to shed light on this aspect and provide a concise and comprehensive answer to the following question:
How do I effectively check for equality of interface{} values, particularly in cases involving user-defined structs?
Interface{} Equality
Firstly, it's essential to understand that interface{} values support equality comparison. Two interface{} values are equal if they have identical dynamic types and equal dynamic values, or if both have a nil value.
Structs and Interfaces
When comparing a struct value to an interface{} value, equality holds true only if values of the struct's type are comparable and the struct implements the interface. Furthermore, the struct's dynamic type must match the interface's dynamic type, and its dynamic value must be equal to the interface's dynamic value.
Example
Let's consider an example to illustrate this behavior:
type MyStruct struct { Name string Age int } var v interface{} = MyStruct{Name: "John", Age: 30} var A = []interface{}{MyStruct{Name: "Alice", Age: 25}, MyStruct{Name: "Bob", Age: 35}} for i := 0; i < len(A); i++ { if A[i] == v { fmt.Println("Found") break } }
In this scenario, the equality check between A[i] and v will succeed because both values are of type MyStruct with identical fields.
Conclusion
Equality checking in Go becomes manageable once you grasp the concepts of interface{} equality and the relationship between structs and interfaces. By understanding these principles, you can effectively compare interface{} values, including custom structs, with confidence.
The above is the detailed content of How Can I Compare `interface{}` Values for Equality in Go, Especially with Custom Structs?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


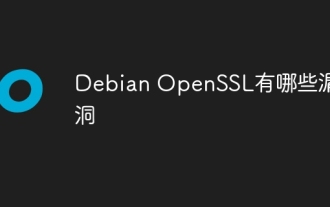
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
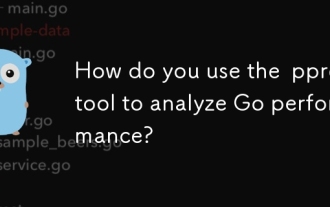
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
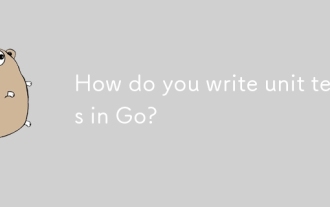
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
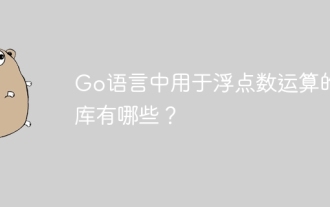
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
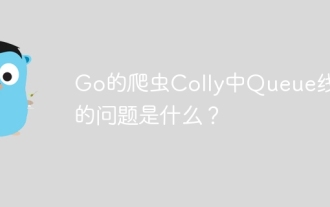
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
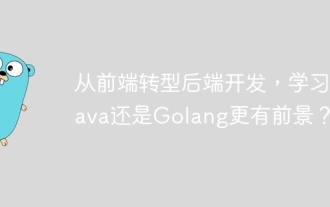
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
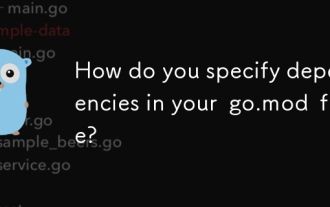
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
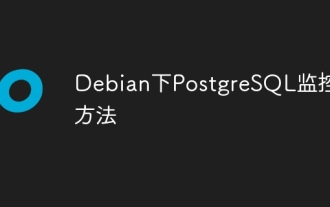
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
