


How to Calculate the Time Difference in Hours and Minutes Between Two Pandas Columns?
Calculate Time Difference Between Two Pandas Columns in Hours and Minutes
When working with time-based data in Pandas, it's often necessary to calculate the difference between two date or datetime columns. By default, this calculation returns a datetime.timedelta object that includes days, hours, minutes, and seconds. However, in certain scenarios, you may only want to display the hours and minutes.
To achieve this, we can leverage the as_type method provided by Pandas. Here's how:
import pandas as pd import numpy as np # Create a DataFrame with 'todate' and 'fromdate' columns data = {'todate': pd.to_datetime(['2014-01-24 13:03:12.050000', '2014-01-27 11:57:18.240000', '2014-01-23 10:07:47.660000']), 'fromdate': pd.to_datetime(['2014-01-26 23:41:21.870000', '2014-01-27 15:38:22.540000', '2014-01-23 18:50:41.420000'])} df = pd.DataFrame(data) # Calculate the difference between 'todate' and 'fromdate' df['diff'] = df['fromdate'] - df['todate'] # Convert the 'diff' column to hours and minutes df['diff'] = df['diff'].astype(np.timedelta64, copy=False)
By converting the diff column to a timedelta64 object with a precision of hours, we disregard the days component and retain only the hours and minutes.
Output:
todate fromdate diff 0 2014-01-24 13:03:12.050 2014-01-26 23:41:21.870 58 hours 0 minutes 1 2014-01-27 11:57:18.240 2014-01-27 15:38:22.540 3 hours 41 minutes 2 2014-01-23 10:07:47.660 2014-01-23 18:50:41.420 8 hours 42 minutes
The above is the detailed content of How to Calculate the Time Difference in Hours and Minutes Between Two Pandas Columns?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










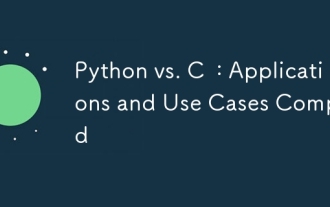
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
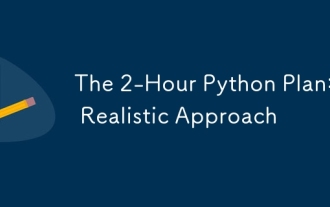
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
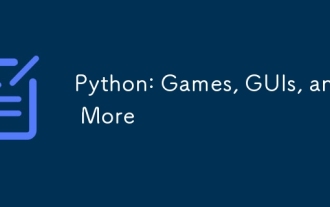
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
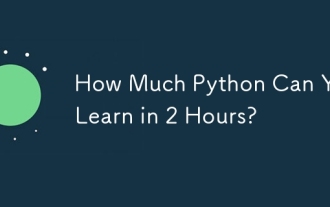
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
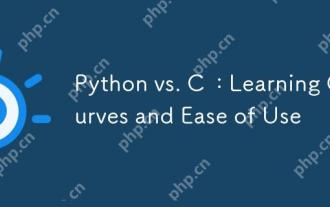
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
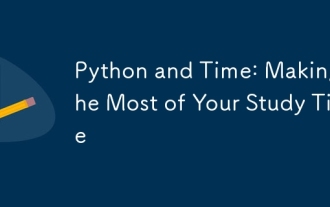
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
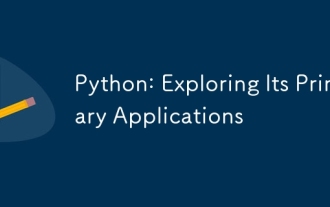
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
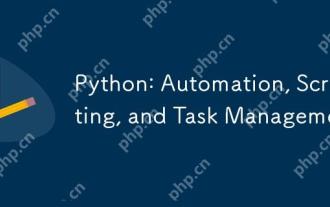
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
