


How to Sort a Multidimensional PHP Array by a Nested Field Efficiently?
How to Efficiently Sort a Multidimensional PHP Array by a Nested Field?
In PHP, it is possible to encounter multidimensional arrays representing tabular data, where each element holds an associative array of field-value pairs. The task of sorting this data by a specific field within the nested arrays can be achieved with ease.
Consider the following database-alike array:
$data = [ [ 'name' => 'Sony TV', 'price' => 600.00 ], [ 'name' => 'LG TV', 'price' => 350.00 ], [ 'name' => 'Samsung TV', 'price' => 425.00 ] ];
To sort this array by the 'price' field, one can employ the array_multisort() function along with the array_column() function, which extracts a specific column (field) from the multidimensional array. The following snippet accomplishes this:
array_multisort(array_column($data, 'price'), SORT_ASC, $data);
This call will rearrange the array in ascending order based on the 'price' field while discarding the original outer array keys. The resulting sorted array will appear as:
[ [ 'name' => 'LG TV', 'price' => 350.00 ], [ 'name' => 'Samsung TV', 'price' => 425.00 ], [ 'name' => 'Sony TV', 'price' => 600.00 ] ]
Alternatively, for PHP versions before 8, a two-liner solution was required due to reference passing restrictions:
$col = array_column($data, 'price'); array_multisort($col, SORT_ASC, $data);
The above is the detailed content of How to Sort a Multidimensional PHP Array by a Nested Field Efficiently?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










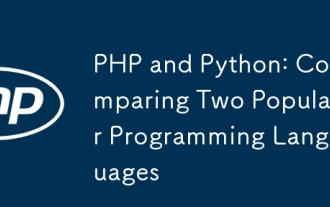
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
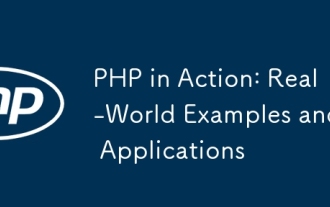
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
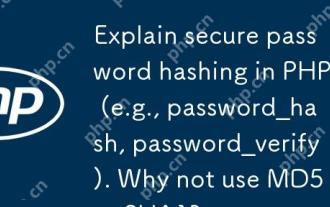
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
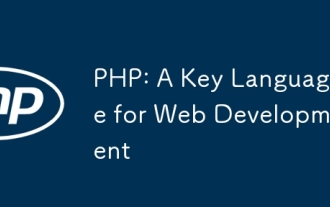
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
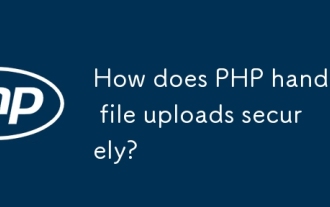
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
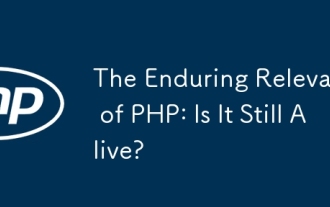
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
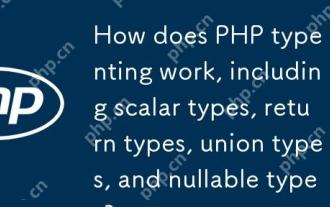
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
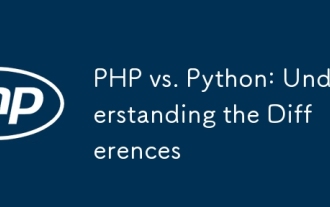
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
