How Can I Efficiently Get a File's Extension in Java?
Dec 02, 2024 am 04:41 AMObtaining File Extensions in Java
If you're looking to retrieve the file extension of a path in Java, you may wonder if there's a straightforward built-in method to do so. While Java doesn't offer a native solution, leveraging Apache Commons IO's FilenameUtils.getExtension method provides a simple and effective alternative.
To utilize FilenameUtils.getExtension, specify the file's full path or its name only. For instance:
import org.apache.commons.io.FilenameUtils; String ext1 = FilenameUtils.getExtension("/path/to/file/foo.txt"); // "txt" String ext2 = FilenameUtils.getExtension("bar.exe"); // "exe"
In order to use FilenameUtils.getExtension, you'll need to include the Apache Commons IO dependency in your project:
Maven:
<dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.6</version> </dependency>
Gradle Groovy DSL:
implementation 'commons-io:commons-io:2.6'
Gradle Kotlin DSL:
implementation("commons-io:commons-io:2.6")
Alternatively, you can refer to the Maven Central repository (https://search.maven.org/artifact/commons-io/commons-io/2.6/jar) for other dependency management options.
The above is the detailed content of How Can I Efficiently Get a File's Extension in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
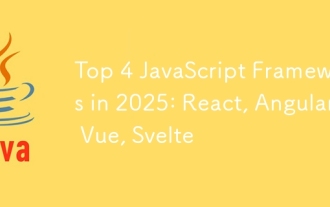
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
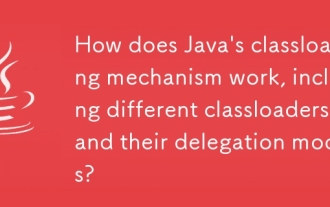
How does Java's classloading mechanism work, including different classloaders and their delegation models?
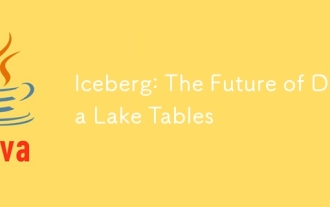
Iceberg: The Future of Data Lake Tables
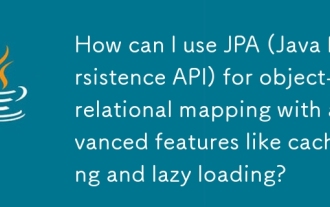
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
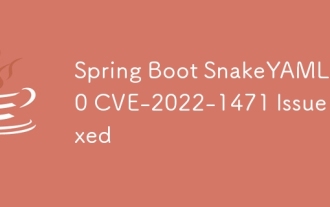
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
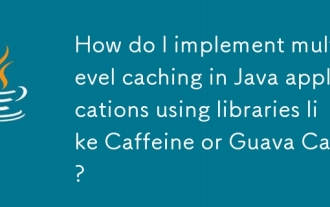
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
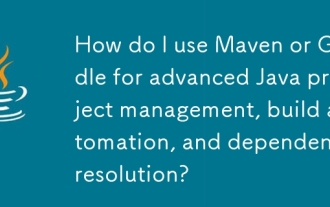
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
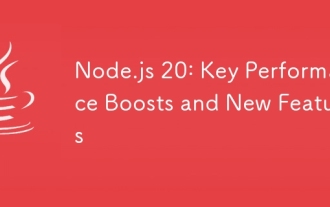
Node.js 20: Key Performance Boosts and New Features
