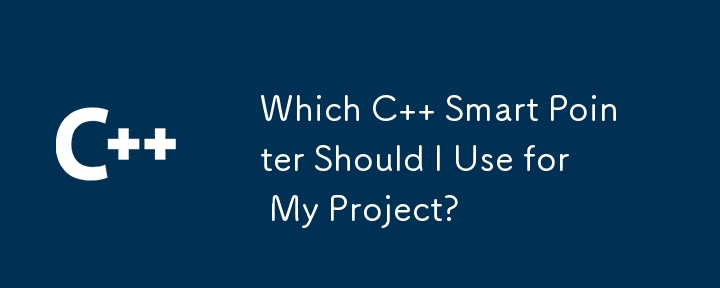
Which C Smart Pointers to Use: A Comprehensive Comparison
Introduction: Exploring the Landscape of C Smart Pointers
In the realm of modern C programming, smart pointers are essential tools for managing dynamically allocated memory and ensuring resource safety. With their ability to automatically handle object lifetime, smart pointers enhance code quality, minimize memory leaks, and simplify cleanup. However, with a vast array of smart pointer implementations available, selecting the right one for each scenario can be a challenge. This discussion analyzes and compares the prominent smart pointer implementations in C to guide you in making informed decisions.
C C 03 Implementations
std::auto_ptr: Although generally considered as a pioneer in smart pointer development, std::auto_ptr falls short with its limited garbage collection capabilities and drawbacks such as unsafe handling of arrays and incompatibility with STL containers. Its pending deprecation further discourages its use.std::auto_ptr_ref: Not a true smart pointer but a complementary construct, std::auto_ptr_ref assists in creating copyable and assignable std::auto_ptr instances through the Colvin-Gibbons trick.
C C 11 Implementations
std::unique_ptr: As the successor to std::auto_ptr, std::unique_ptr provides superior functionality by addressing the shortcomings of its predecessor. It supports array management, ownership protection, and compatibility with STL containers.std::shared_ptr: Derived from TR1 and boost implementations, std::shared_ptr embodies a reference-counted smart pointer. Its key feature is shared ownership, enabling multiple pointers to a single object. Automatic deletion occurs when the reference count drops to zero, ensuring memory consistency.std::weak_ptr: Accompanying std::shared_ptr, std::weak_ptr grants a non-owning reference to an object. Its primary value lies in preventing dangling references and circular dependencies.
Boost Implementations
boost::shared_ptr: Widely regarded as the gold standard for shared smart pointers, boost::shared_ptr offers broad applicability in various scenarios. Despite potential performance concerns, it remains a popular choice.boost::weak_ptr: Similar to std::weak_ptr, boost::weak_ptr complements boost::shared_ptr, providing non-owning references.boost::scoped_ptr: Resembling std::auto_ptr, boost::scoped_ptr is a simple, fast smart pointer primarily intended for exclusive ownership scenarios.boost::intrusive_ptr: Designed for use with custom smart pointer compatible classes, boost::intrusive_ptr provides flexibility but requires user-managed reference counting and may pose thread safety challenges.boost::shared_array: An array-oriented version of boost::shared_ptr, boost::shared_array offers STL compatibility and built-in array functionality.boost::scoped_array: Similar to boost::scoped_ptr, boost::scoped_array focuses on arrays, eliminating the need for std::vector in most cases.
Qt Implementations
QPointer: A weak pointer restricted to QObject and its derivatives, QPointer carries limitations with object lifetime checks and potential issues in multi-threaded environments.QSharedDataPointer: A strong pointer comparable to boost::intrusive_ptr, QSharedDataPointer necessitates custom reference management via QSharedData subclassing.QExplicitlySharedDataPointer: Similar to QSharedDataPointer but with greater control over detaching after reference count drops to zero.QSharedPointer: A reference-counted, thread-safe, and versatile pointer, QSharedPointer offers shared ownership capabilities in Qt environments.QWeakPointer: Qt's companion weak pointer, QWeakPointer facilitates non-owning references to QSharedPointer objects.QScopedPointer: Heavily inspired by boost::scoped_ptr, QScopedPointer provides exclusive ownership without the overhead of QSharedPointer.
Conclusion
Selecting the appropriate C smart pointer relies on understanding their respective strengths, weaknesses, and applicability. For exclusive ownership scenarios, std::unique_ptr or boost::scoped_ptr are ideal. For shared ownership, std::shared_ptr, boost::intrusive_ptr, or Qt's QSharedPointer are strong choices. Non-owning references are effectively handled by std::weak_ptr, boost::weak_ptr, and their Qt counterparts. Remember, tailoring
The above is the detailed content of Which C Smart Pointer Should I Use for My Project?. For more information, please follow other related articles on the PHP Chinese website!