


Why Does Modifying a Variable in a `finally` Block Not Affect the Return Value in Java?
Why does modifying a variable in a finally block not affect the return value?
In Java, it's generally understood that the finally block should not affect the return value of the method. However, it may be confusing why this is the case, especially when the return value is modified within the finally block.
Consider the following example:
public class Test { private String s; public String foo() { try { s = "dev"; return s; } finally { s = "override variable s"; System.out.println("Entry in finally Block"); } } public static void main(String[] xyz) { Test obj = new Test(); System.out.println(obj.foo()); } }
The output of this code is:
Entry in finally Block dev
Why is s not overridden in the finally block, yet the control printed output?
Explanation
The try block completes with the execution of the return statement. The value of s at the time the return statement executes is the value returned by the method. The fact that the finally clause later changes the value of s (after the return statement completes) does not (at that point) change the return value.
In the example above, the return statement in the try block sets the return value to "dev". The finally block then sets s to "override variable s", but this change occurs after the return value has already been set. Therefore, the return value is still "dev".
It's important to note that this behavior only applies to changes in the value of primitive variables or immutable objects. If s were a reference to a mutable object and the contents of the object were changed in the finally block, then those changes would be seen in the returned value.
The above is the detailed content of Why Does Modifying a Variable in a `finally` Block Not Affect the Return Value in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










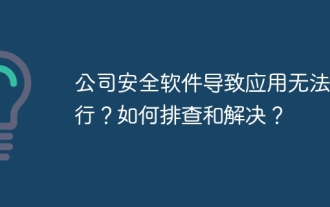
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
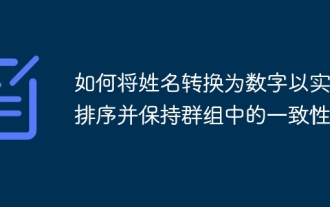
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
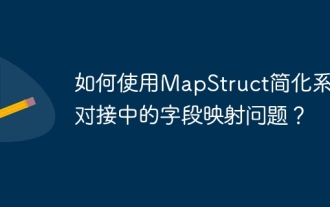
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
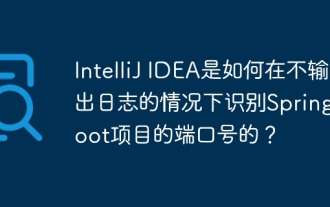
Start Spring using IntelliJIDEAUltimate version...
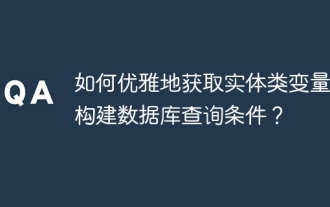
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
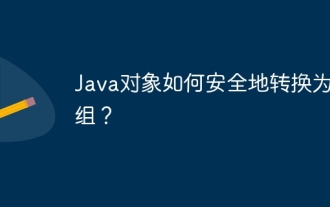
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
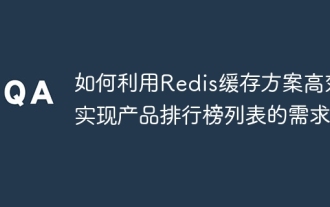
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
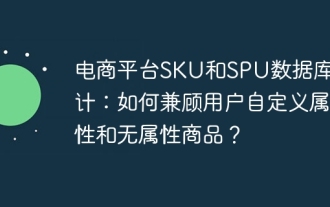
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
