How Can I Achieve Exclusive File Locking in Go?
Locking Files Exclusively in Go
Cross-process file locking is essential for ensuring that multiple processes don't interfere with each other while accessing the same file. In .NET, this can be achieved using File.Open with FileShare.None. How can we achieve exclusive file access in Go?
Solution: Using the fslock Package
The fslock package provides a cross-platform solution for file locking. It utilizes LockFileEx on Windows and flock on *nix systems.
Getting Started with fslock
To use fslock, begin by creating a new lock object:
lock := fslock.New("file.txt")
This command creates the lock file if it doesn't already exist.
Acquiring a Lock
To acquire an exclusive lock on the file, use the Lock method:
lockErr := lock.Lock() if lockErr != nil { // Handle error }
Alternatively, you can use LockWithTimeout to wait for the lock within a specified duration:
lockErr := lock.LockWithTimeout(10 * time.Second) if lockErr != nil { // Handle timeout }
Releasing the Lock
When you're done with the file, release the lock using Unlock:
lock.Unlock()
Example Usage
The following code snippet demonstrates how to use the fslock package to lock a file for exclusive access:
package main import ( "time" "fmt" "github.com/juju/fslock" ) func main() { lock := fslock.New("file.txt") lockErr := lock.TryLock() if lockErr != nil { fmt.Println("Failed to acquire lock:", lockErr) return } fmt.Println("Got the lock") time.Sleep(1 * time.Minute) // Release the lock lock.Unlock() }
The above is the detailed content of How Can I Achieve Exclusive File Locking in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










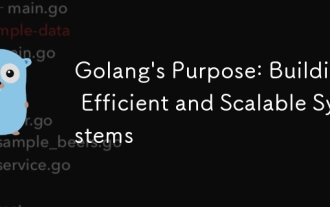
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
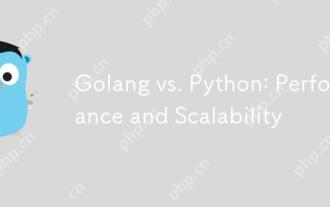
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
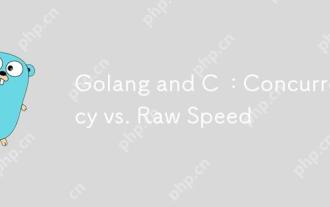
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
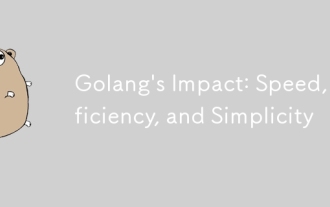
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
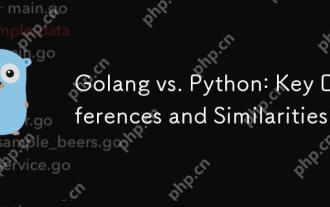
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
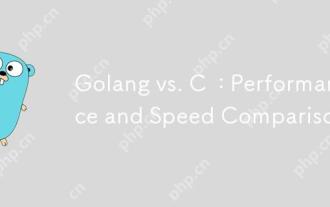
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
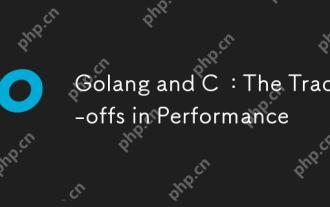
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
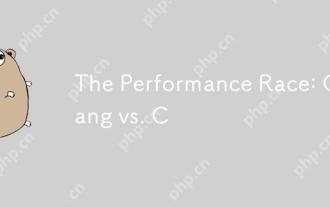
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
