


How Can I Efficiently Sort Collections of Objects by Multiple Properties in Java?
Sorting Collections of Objects
As a developer, you may often encounter situations where you need to sort collections of objects based on different criteria. When dealing with simple data types like strings, sorting is straightforward using functions like Collections.sort(). However, when working with custom classes like Person, sorting becomes more complex.
Here's an efficient solution to sorting a collection of objects by multiple properties:
Implement a Custom Comparator Interface
The Comparator
For example, consider a Person class with properties such as name, age, and country. To enable sorting by these properties, create a FlexiblePersonComparator class:
import java.util.Comparator; public class FlexiblePersonComparator implements Comparator<Person> { // Define sorting order options as an enum public enum Order {Name, Age, Country} private Order sortingBy = Name; @Override public int compare(Person person1, Person person2) { switch (sortingBy) { case Name: return person1.name.compareTo(person2.name); case Age: return person1.age.compareTo(person2.age); case Country: return person1.country.compareTo(person2.country); } // Add a fail-safe runtime exception to avoid unreachable code throw new RuntimeException("Practically unreachable code, can't be thrown"); } // Expose a method to set the sorting order public void setSortingBy(Order sortBy) { this.sortingBy = sortBy; } }
Using the Custom Comparator
Once the custom comparator is defined, you can create an instance and specify the desired sorting order. Then, use this instance to sort the collection of objects:
public void sortPersonsBy(FlexiblePersonComparator.Order sortingBy) { List<Person> persons = this.persons; FlexiblePersonComparator comparator = new FlexiblePersonComparator(); comparator.setSortingBy(sortingBy); Collections.sort(persons, comparator); }
By passing in the desired sorting order, you can easily sort the collection by any of the specified properties, providing flexibility and control over the sorting behavior.
The above is the detailed content of How Can I Efficiently Sort Collections of Objects by Multiple Properties in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










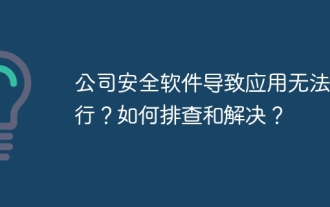
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
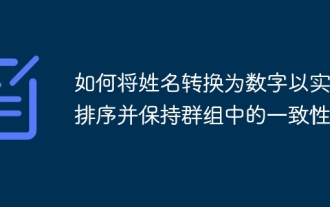
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
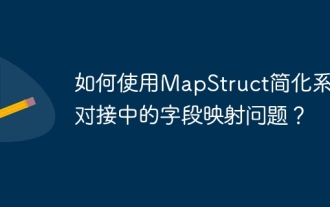
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
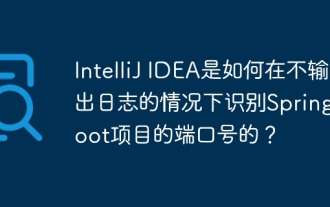
Start Spring using IntelliJIDEAUltimate version...
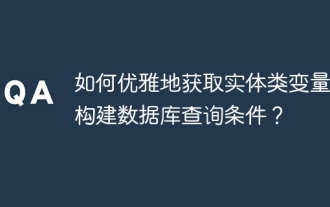
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
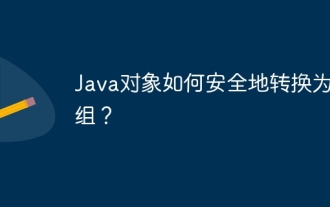
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
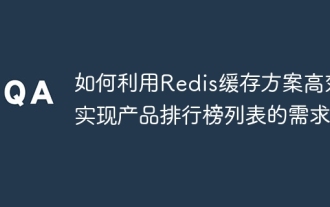
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
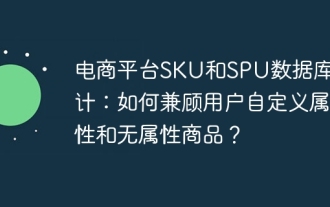
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
