


Why Am I Getting Fetch Errors During Next.js Static Site Compilation with `getStaticProps` and `getStaticPaths`?
Uncovering the Cause of Fetch Errors in Next.js Static Site Compilation
When using Next.js to construct a static website, it's imperative to know the source of certain errors that might arise during the build process. In this instance, we'll address the specific problem faced during npm run build when utilizing both getStaticProps and getStaticPaths to retrieve data from an API route.
Error Details
The primary error being encountered is associated with an invalid JSON response received from the API route when executing npm run build. This response appears to originate from the API route pages/api/products/[slug].js.
Possible Causes
- Incorrect API Routing: Inspect the implementation of pages/api/products/[slug].js thoroughly to ascertain whether the data is fetched and processed appropriately. If there are any mistakes in this code, they may cause the invalid JSON response.
- API Route Availability: Keep in mind that API routes are not accessible during the build process. Therefore, if your getStaticProps or getStaticPaths functions rely on API routes to obtain data, this can lead to errors.
Recommended Resolution
To rectify this issue, consider refactoring your code in the following manner:
- Utilize Server-Side Logic: Since getStaticProps operates exclusively on the server-side, you can bypass API routes by integrating the server-side logic directly into these functions. This eliminates the need to fetch data from an API route, and instead permits you to retrieve data directly.
- Data Retrieval from Server: Directly access the data source, which in this case is stored in data/products, within getStaticProps and getStaticPaths. Modify your functions as follows:
// /pages/product/[slug] import db from '../../../data/products'; // Remaining code. export const getStaticProps = async ({ params: { slug }, locale }) = { const result = db.filter((item) => item.slug === slug); const data = result.filter((item) => item.locale === locale)[0]; const { title, keywords, description } = data; return { props: { data, description, keywords, title, }, }; }; export const getStaticPaths = async () = { const paths = db.map(({ slug, locale }) => ({ params: { slug: slug }, locale, })); return { fallback: true, paths, }; };
By adopting these changes, you'll remove the reliance on the API route, resolve the invalid JSON response error, and allow for seamless static website compilation.
The above is the detailed content of Why Am I Getting Fetch Errors During Next.js Static Site Compilation with `getStaticProps` and `getStaticPaths`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


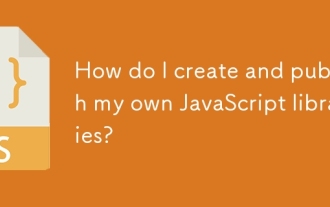
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
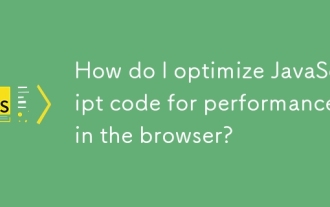
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
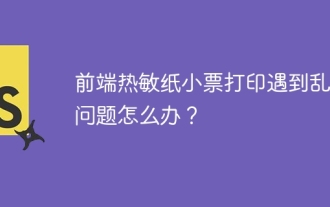
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
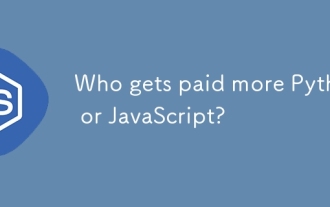
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
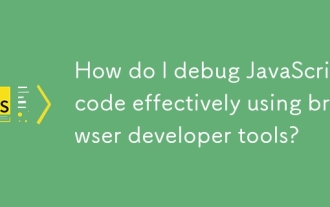
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
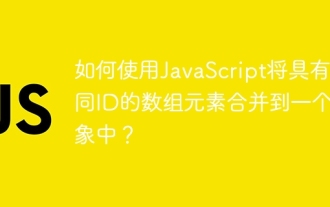
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
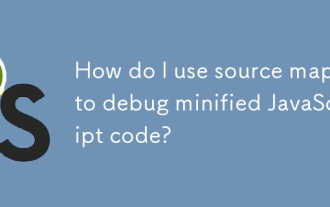
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
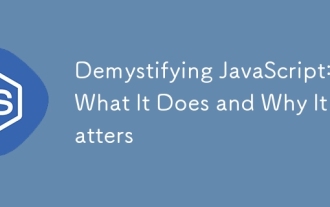
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
