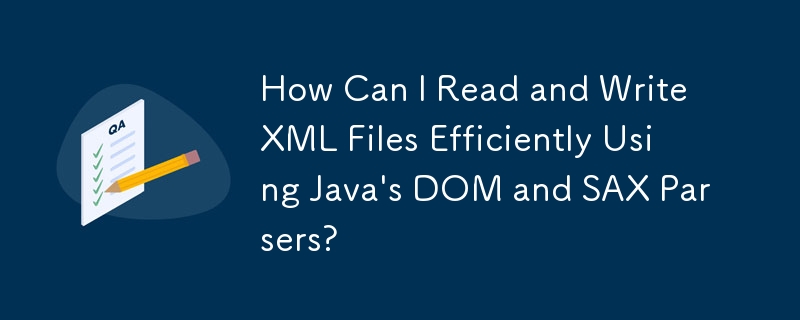
Reading and Writing XML Files Using Java
Introduction
Managing XML data is a common task in Java applications. This article provides a comprehensive explanation of how to read and write XML files using the various built-in Java libraries like DOM and SAX.
DOM: Reading an XML File
-
Import libraries: Import necessary DOM libraries.
import javax.xml.parsers.*;
import org.w3c.dom.*;
Copy after login
Copy after login
-
Create a Document Builder Factory: Instantiate a DocumentBuilderFactory object to create a DocumentBuilder.
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
Copy after login
Copy after login
Copy after login
-
Create a Document Builder: Use the DocumentBuilderFactory to create a DocumentBuilder for parsing XML.
DocumentBuilder db = dbf.newDocumentBuilder();
Copy after login
Copy after login
Copy after login
-
Parse XML: Parse the XML file using the DocumentBuilder.
Document dom = db.parse(xmlFilePath);
Copy after login
Copy after login
-
Get the Root Element: Obtain the root element of the XML document.
Element rootElement = dom.getDocumentElement();
Copy after login
-
Navigate and Extract Data: Traverse the XML structure using the rootElement to access and extract data.
DOM: Writing an XML File
-
Import libraries: Import necessary DOM libraries.
import javax.xml.parsers.*;
import javax.xml.transform.*;
import javax.xml.transform.dom.*;
import javax.xml.transform.stream.*;
Copy after login
-
Create a Document Builder Factory: Instantiate a DocumentBuilderFactory object to create a DocumentBuilder.
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
Copy after login
Copy after login
Copy after login
-
Create a Document Builder: Use the DocumentBuilderFactory to create a DocumentBuilder for creating XML.
DocumentBuilder db = dbf.newDocumentBuilder();
Copy after login
Copy after login
Copy after login
-
Create a New Document: Create a new XML document.
Document dom = db.newDocument();
Copy after login
-
Create Root Element: Add the root element to the document.
Element rootElement = dom.createElement("rootElement");
dom.appendChild(rootElement);
Copy after login
-
Add Data to the XML: Create child elements and append data to the XML structure.
-
Transform and Write to File: Use a Transformer to serialize the XML document and write it to a file.
Transformer tr = TransformerFactory.newInstance().newTransformer();
tr.transform(new DOMSource(dom), new StreamResult(new FileOutputStream("output.xml")));
Copy after login
SAX: Reading and Parsing XML
-
Import libraries: Import necessary SAX libraries.
import javax.xml.parsers.*;
import org.w3c.dom.*;
Copy after login
Copy after login
-
Create a SAX Parser: Create a SAXParserFactory and instantiate a SAXParser.
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
Copy after login
Copy after login
Copy after login
-
Implement ContentHandler: Define a ContentHandler class to handle SAX events.
DocumentBuilder db = dbf.newDocumentBuilder();
Copy after login
Copy after login
Copy after login
-
Parse XML: Parse the XML file using the SAXParser and provide your ContentHandler implementation.
Document dom = db.parse(xmlFilePath);
Copy after login
Copy after login
-
Process SAX Events: In the ContentHandler implementation, process the SAX events to extract and process data.
Additional Resources
- [Java XML Parsing Tutorial](https://www.tutorialspoint.com/javaxml/java_xml_parsing.htm)
- [DOM Tutorial](https://www.w3schools.com/xml/dom_intro.asp)
- [SAX Tutorial](https://www.w3schools.com/xml/sax_intro.asp)
The above is the detailed content of How Can I Read and Write XML Files Efficiently Using Java's DOM and SAX Parsers?. For more information, please follow other related articles on the PHP Chinese website!