How to Properly Copy C 11 Classes Containing `unique_ptr` Members?
Copying Classes with Unique Pointers in C 11
Creating a copy constructor for a class containing a unique_ptr, a smart pointer that enforces exclusive ownership, poses unique challenges. In C 11, managing unique_ptr members requires careful consideration.
Solution:
To implement a copy constructor, you have two options:
- Deep Copy: Create a new copy of the unique_ptr's content. This ensures both objects own their data independently.
class A { std::unique_ptr<int> up_; public: A(int i) : up_(new int(i)) {} A(const A& a) : up_(new int(*a.up_)) {} };
- Convert to shared_ptr: Convert the unique_ptr to a shared_ptr, which allows multiple owners.
std::shared_ptr<int> sp = std::make_shared<int>(*up_);
Additional Considerations:
- Move Constructor: Instead of a copy constructor, you could use a move constructor, which transfers ownership of the unique_ptr.
A(A&& a) : up_(std::move(a.up_)) {}
- Overloading Other Operators: For a complete set of operations, it's helpful to overload the assignment operators.
A& operator=(const A& a) { up_.reset(new int(*a.up_)); return *this; } A& operator=(A&& a) { up_ = std::move(a.up_); return *this; }
- Vector Considerations: If you intend to use your class in a std::vector, decide if the vector will exclusively own the objects. You can enforce move-only behavior by omitting the copy constructor and copy assignment operators.
The above is the detailed content of How to Properly Copy C 11 Classes Containing `unique_ptr` Members?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


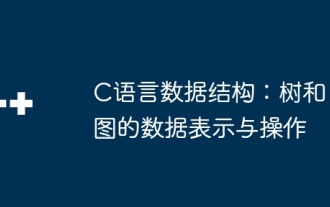
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
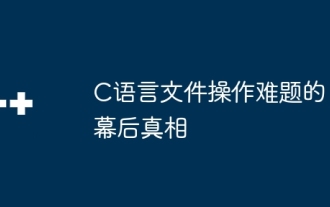
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
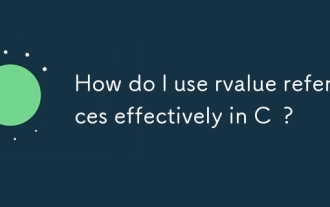
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
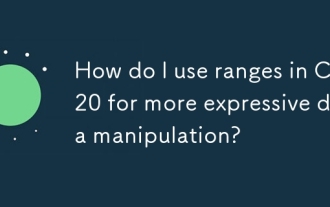
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
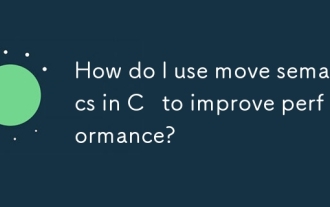
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
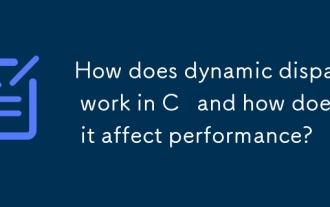
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
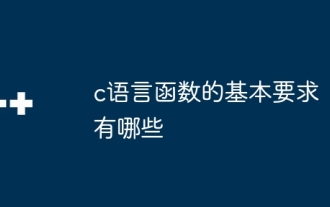
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
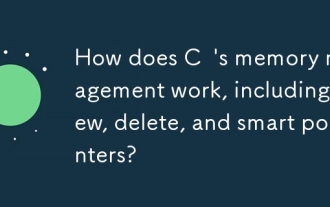
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
