


How Can I Efficiently Check for List Element Equality in Python?
Simplifying and Accelerating List Element Equality Checks
Background Information
Python provides convenient mechanisms to check whether all elements in a list satisfy a specific condition. Existing approaches utilize the built-in function all() to perform this task efficiently. Additionally, for conditions involving membership in another container, optimized solutions are available.
Using all() for Equality Verification
The simplest and fastest method to check if all elements of a list match a condition is to employ the all() function. This function evaluates if the condition holds true for every element in the sequence. For example, to ascertain whether each last element in a sublist is 0:
import all my_list = [[1, 2, 3], [4, 5, 0], [7, 8, 0]] result = all(item[2] == 0 for item in my_list) print(result) # True
Leveraging Generator Expression for Efficiency
To further enhance efficiency, generator expressions can be combined with all(). This combination generates the elements in the list lazily, providing a streamlined evaluation process.
result = all(flag == 0 for (_, _, flag) in my_list)
Utilizing any() for Inequality Verification
Conversely, to check if at least one element of a list matches a condition, any() can be employed. This function determines whether any element in the sequence satisfies the condition.
result = any(flag == 0 for (_, _, flag) in my_list)
Alternative Approaches for Element Filtering
In scenarios where an element needs to be filtered based on a condition, list comprehensions offer an effective solution:
filtered_list = [x for x in my_list if x[2] == 0]
This comprehension extracts all sublists where the last element is 0. Similarly, one can use filter():
filtered_list = filter(lambda x: x[2] == 0, my_list)
The above is the detailed content of How Can I Efficiently Check for List Element Equality in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










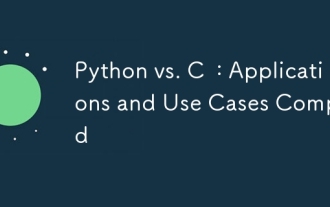
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
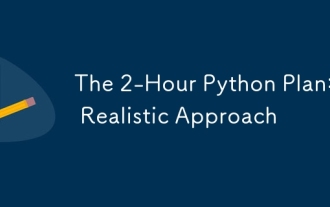
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
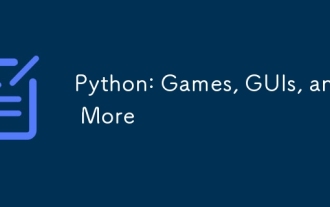
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
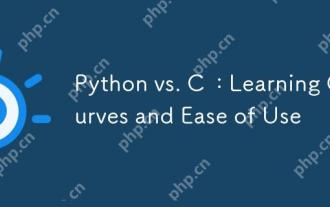
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
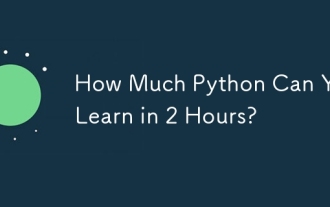
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
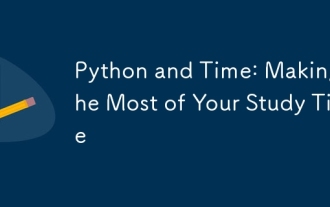
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
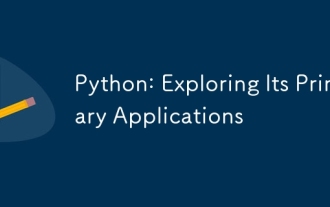
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
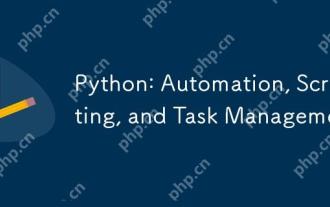
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
