


How to Achieve the Equivalent of PHP\'s htmlspecialchars in JavaScript?
JavaScript Equivalent to PHP's htmlspecialchars
In JavaScript, there is no built-in function directly analogous to PHP's htmlspecialchars for converting HTML special characters to character entities. However, there are custom solutions that can achieve a similar result.
Custom Implementation
One approach is to define a custom function that manually translates each special character. For instance:
function escapeHtml(text) { return text .replace(/&/g, "&") .replace(/</g, "<") .replace(/>/g, ">") .replace(/"/g, """) .replace(/'/g, "'"); }
This function takes a string as input and replaces all occurrences of the following characters with their corresponding character entities:
- & - &
- < - <
- >
- " - "
- ' - '
Improved Performance
For better performance, especially with large texts, you can use a slightly optimized version:
function escapeHtml(text) { var map = { '&': '&', '<': '<', '>': '>', '"': '"', "'": ''' }; return text.replace(/[&<>"']/g, function(m) { return map[m]; }); }
Copy after loginThis version uses a lookup table to store the character entity mappings, which eliminates the need for multiple replace operations.
The above is the detailed content of How to Achieve the Equivalent of PHP\'s htmlspecialchars in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
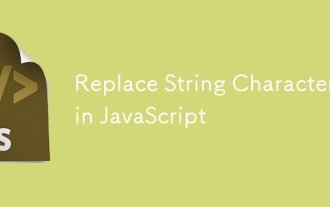
Replace String Characters in JavaScript
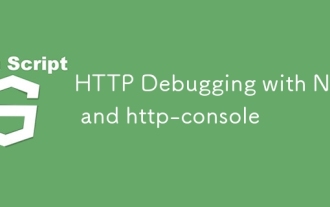
HTTP Debugging with Node and http-console
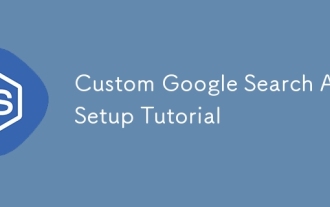
Custom Google Search API Setup Tutorial
