How Should You Properly Reuse Moved Containers in C ?
Dec 03, 2024 am 02:42 AMReusing Moved Containers: The Right Approach
The topic of reusing moved containers in C has sparked discussions. This article explores the correct method for handling such situations.
Consider the following code snippet:
std::vector<int> container; container.push_back(1); auto container2 = std::move(container); // ver1: Do nothing //container2.clear(); // ver2: "Reset" container = std::vector<int>() // ver3: Reinitialize container.push_back(2); assert(container.size() == 1 && container.front() == 2);
According to the C 0x standard draft, ver3 appears to be the preferred approach since a moved object resides in "a valid but unspecified state." To clarify this concept, let's refer to the definition in the standard:
[defns.valid] §17.3.26 valid but unspecified state an object state that is not specified except that the object’s invariants are met and operations on the object behave as specified for its type
This means that the moved object is still alive and can be operated upon as usual, excluding actions with preconditions (unless the precondition is verified beforehand).
For instance, the clear() operation has no preconditions. By employing clear(), the container is reset to a defined state, enabling its subsequent utilization.
Consequently, the correct way to reuse a moved container is to perform a clear() operation, as demonstrated in ver2 in the code snippet. This approach ensures that the container is restored to a known and consistent state.
The above is the detailed content of How Should You Properly Reuse Moved Containers in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
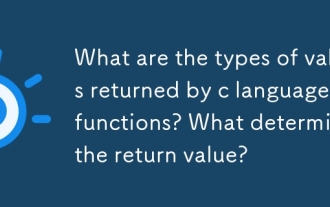
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
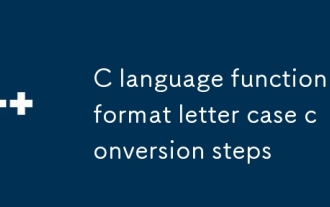
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
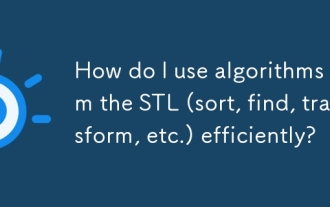
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
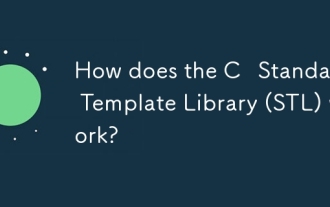
How does the C Standard Template Library (STL) work?
