How Can Buffered Channels Improve Concurrency in Go?
Understanding the Use of Buffered Channels in Go
Buffered channels, unlike their synchronous counterparts, allow for a specific buffer size to be defined when created. This provides several benefits and is often used in situations where multiple parallel actions are required.
In the example provided:
package main import "fmt" import "time" func longLastingProcess(c chan string) { time.Sleep(2000 * time.Millisecond) c <- "tadaa" } func main() { c := make(chan string) go longLastingProcess(c) go longLastingProcess(c) go longLastingProcess(c) fmt.Println(<-c) }
Each goroutine running longLastingProcess eventually sends a message to the channel c. Without a buffer, only the first message will be received immediately, while the others will block until the first message is consumed.
Practical Uses of Buffered Channels:
Buffered channels become valuable when you need to decouple the producer and consumer processes, such as in the following use cases:
- Task Queuing: A channel with a buffer size greater than 1 can function as a task queue. The producer (scheduler) can deposit jobs into the queue without blocking, allowing the consumer (worker) to process them at its own pace.
- Data Buffering: When working with large datasets, a buffered channel can act as a temporary buffer. The producer (data source) can send data into the channel, while the consumer (data processor) reads from the buffer, potentially at a slower rate, without causing the producer to block.
- Communication between Services: In a distributed system, buffered channels can be used for communication between different services. The buffer provides a temporary storage for messages, allowing services to maintain a steady flow of messages even if they experience temporary delays or outages.
By increasing the buffer size, you can mitigate the risk of channel blocking and ensure smoother communication between the producer and consumer processes. It's worth noting that, while buffered channels offer flexibility, careful consideration is required to avoid over-buffering, which can lead to resource exhaustion or performance issues.
The above is the detailed content of How Can Buffered Channels Improve Concurrency in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










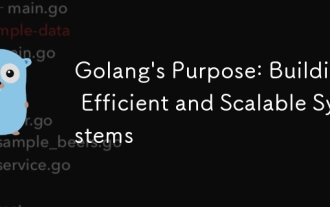
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
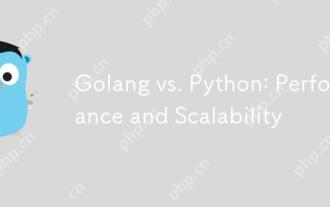
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
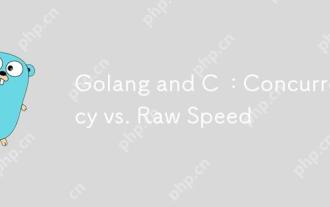
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
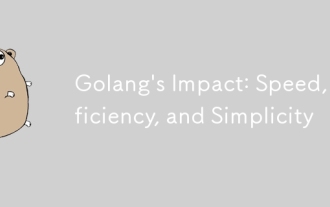
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
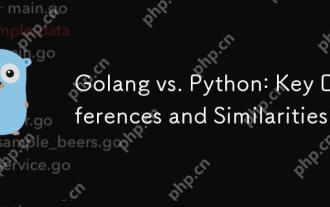
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
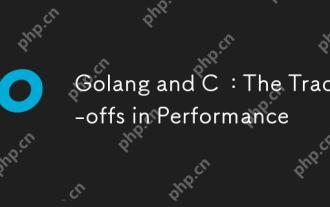
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
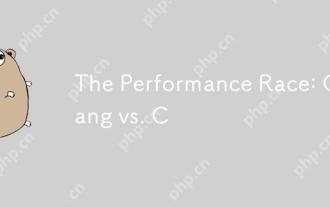
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
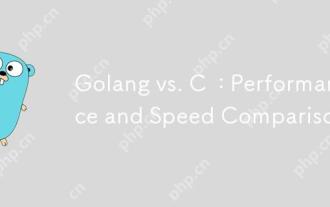
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
