


How Can I Update a Parent Component's State from a Nested Child Component in React without Using Redux?
Updating a Parent's State in React without Redux
Many developers encounter the challenge of communicating state between nested components. A common scenario arises when a child component needs to update the state of a higher-level parent component. This becomes problematic since React dictates that props are immutable.
Consider the following component structure:
Component 1 - Component 2 - Component 4 - Component 5 Component 3
In this scenario, Component 3 requires access to state stored in Component 5. It may seem intuitive to pass the state of Component 5 as a prop to Component 1 and forward it to the other components. However, React's immutability rules prohibit this approach.
One solution to this problem is to implement child-parent communication using a function provided by the parent component. Consider the following code snippet:
class Parent extends React.Component { constructor(props) { super(props) this.handler = this.handler.bind(this) } handler() { this.setState({ someVar: 'some value' }) } render() { return <Child handler={this.handler} /> } } class Child extends React.Component { render() { return <Button onClick={this.props.handler}/> } }
In this example, the handler function provided by the Parent component is passed to the Child component as a prop. When the button in the Child component is clicked, it invokes the handler function, updating the state of the Parent component.
It's important to note that this solution requires restructuring the component hierarchy to ensure logical relationships between components. In the given example, Component 5 and Component 3 may not be directly related. Therefore, it may be necessary to create a new component that encompasses them, allowing for the state to be managed effectively.
The above is the detailed content of How Can I Update a Parent Component's State from a Nested Child Component in React without Using Redux?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


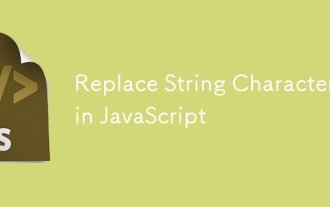
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
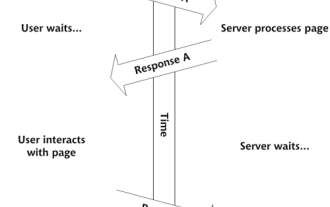
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
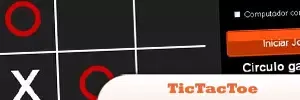
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
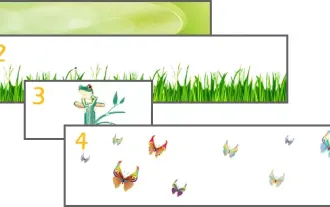
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
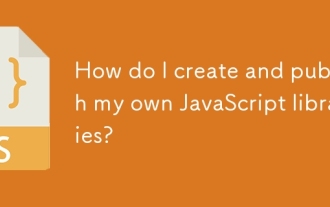
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
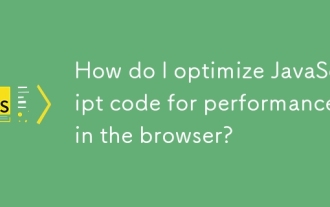
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
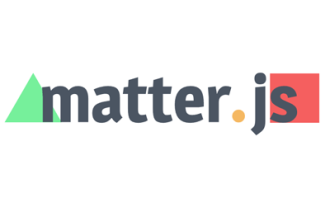
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
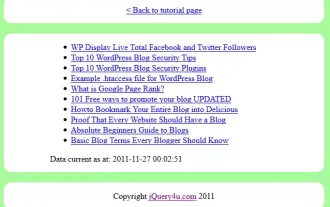
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona
