How to Efficiently Parse an Integer from a Character in Java?
Parsing Integer Values from Characters in Java
Question:
How can you efficiently parse an integer value from a character within a string? Consider that the character at a specific index is known to be a digit.
Initial Approach:
One common approach is to create a String from the character and then convert it to an integer using Integer.parseInt(), as demonstrated in the example:
String element = "el5"; String s = ""+element.charAt(2); int x = Integer.parseInt(s);
Improved Solution:
However, there's a more concise and efficient method using Character.getNumericValue(char). This method accepts a character and returns its numeric value, if the character represents a digit:
String element = "el5"; int x = Character.getNumericValue(element.charAt(2));
This approach eliminates the need to create an intermediate String and results in the same outcome:
x=5
Unicode Support:
One significant advantage of Character.getNumericValue() is its support for Unicode characters representing digits. For instance, it correctly handles characters like "el٥" and "el५," representing the digit 5 in Eastern Arabic and Hindi/Sanskrit, respectively:
String element = "el٥"; int x = Character.getNumericValue(element.charAt(2)); System.out.println("x=" + x);
This example will produce:
x=5
The above is the detailed content of How to Efficiently Parse an Integer from a Character in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










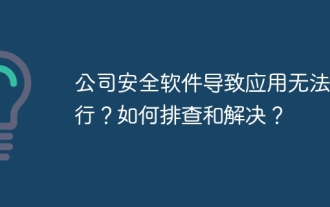
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
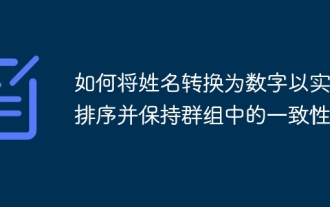
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
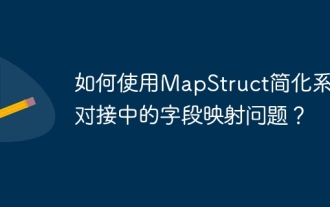
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
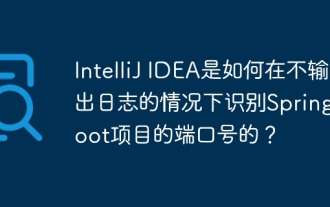
Start Spring using IntelliJIDEAUltimate version...
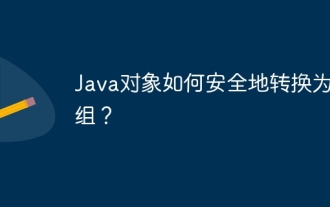
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
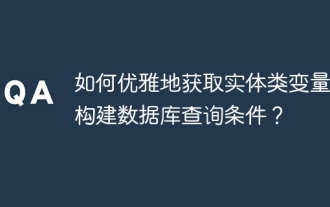
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
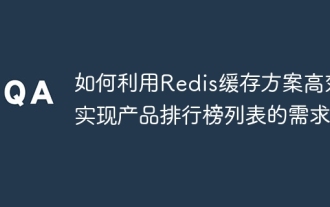
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
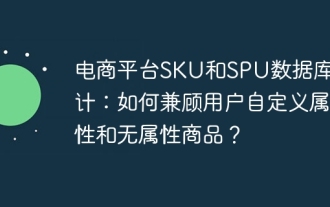
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
