WebSocket with JavaScript and Bun
Ensuring efficient and seamless communication between the client and server is key when building modern, real-time web applications. Traditional HTTP requests—like those used in polling—are stateless and one-directional. The client makes a request (e.g., using fetch or axios) to the server, and the server responds before the connection is closed. If the client needs fresh data, it must repeatedly send new requests at regular intervals, creating unnecessary latency and increasing load on both the client and server.
For example, if you're building a live chat app or a stock price tracker, polling would require the client to request updates every second or so, even when there’s no new data to fetch. This is where WebSockets shine.
The WebSocket approach
WebSockets provide a persistent, two-way communication channel between the client and the server. Once the connection is established, the server can instantly push updates to the client without waiting for a new request. This makes WebSockets ideal for scenarios where real-time updates are essential, such as:
- Sending chat messages in a live chat application.
- Broadcasting notifications or updates to multiple users simultaneously.
- Streaming real-time data, such as stock prices, sports scores, or game states.
Using Vanilla JavaScript on the client side and the Bun runtime on the server side makes implementing WebSockets straightforward and efficient. For example:
- The client can send a message to the server, and the server can instantly broadcast that message to other connected clients.
- A persistent connection ensures no repetitive overhead of re-establishing connections, unlike polling.
In this scenario, WebSockets offer lower latency, reduced server load, and a smoother user experience than traditional polling methods.
Building a WebSocket project
Step 1: setting up a Bun Project
First, ensure the Bun is installed. Then create a new Bun project, create a new empty directory, enter into the new directory, and initialize the project via the bun init command:
mkdir websocket-demo cd websocket-demo bun init
The bun init command will create the package.json file, a "hello world" index.ts file, the .gitignore file, the tsconfig.json file for the typescript configuration, and a README.md file.
Now, you can start creating your JavaScript code. I'm going to show you the whole script; then we will explore all the relevant parts. You can edit the index.ts file:
console.log("? Hello via Bun! ?"); const server = Bun.serve({ port: 8080, // defaults to $BUN_PORT, $PORT, $NODE_PORT otherwise 3000 fetch(req, server) { const url = new URL(req.url); if (url.pathname === "/") return new Response(Bun.file("./index.html")); if (url.pathname === "/surprise") return new Response("?"); if (url.pathname === "/chat") { if (server.upgrade(req)) { return; // do not return a Response } return new Response("Upgrade failed", { status: 400 }); } return new Response("404!"); }, websocket: { message(ws, message) { console.log("✉️ A new Websocket Message is received: " + message); ws.send("✉️ I received a message from you: " + message); }, // a message is received open(ws) { console.log("? A new Websocket Connection"); ws.send("? Welcome baby"); }, // a socket is opened close(ws, code, message) { console.log("⏹️ A Websocket Connection is CLOSED"); }, // a socket is closed drain(ws) { console.log("DRAIN EVENT"); }, // the socket is ready to receive more data }, }); console.log(`? Server (HTTP and WebSocket) is launched ${server.url.origin}`);
Documenting the code for a basic WebSocket server using Bun
Below is a breakdown of the provided code, explaining each part and its functionality.
Server Initialization
mkdir websocket-demo cd websocket-demo bun init
The Bun.serve method initializes a server capable of handling both HTTP and WebSocket requests.
- port: 8080: Specifies the port on which the server listens. Defaults to common environment variables or 3000 if unspecified. In this example the port is hardcoded to 8080. If you want to provide a more flexible way, you should remove the port line and allow to Bun to manage the port. So you can run the script via export BUN_PORT=4321; bun run index.ts
HTTP request handling
console.log("? Hello via Bun! ?"); const server = Bun.serve({ port: 8080, // defaults to $BUN_PORT, $PORT, $NODE_PORT otherwise 3000 fetch(req, server) { const url = new URL(req.url); if (url.pathname === "/") return new Response(Bun.file("./index.html")); if (url.pathname === "/surprise") return new Response("?"); if (url.pathname === "/chat") { if (server.upgrade(req)) { return; // do not return a Response } return new Response("Upgrade failed", { status: 400 }); } return new Response("404!"); }, websocket: { message(ws, message) { console.log("✉️ A new Websocket Message is received: " + message); ws.send("✉️ I received a message from you: " + message); }, // a message is received open(ws) { console.log("? A new Websocket Connection"); ws.send("? Welcome baby"); }, // a socket is opened close(ws, code, message) { console.log("⏹️ A Websocket Connection is CLOSED"); }, // a socket is closed drain(ws) { console.log("DRAIN EVENT"); }, // the socket is ready to receive more data }, }); console.log(`? Server (HTTP and WebSocket) is launched ${server.url.origin}`);
- fetch(req, server): Handles incoming HTTP requests.
- Root path /: serves the index.html file.
- /surprise path: returns a fun surprise emoji response ?.
- /chat Path: Tries to "upgrade" the connection to a WebSocket connection. If the upgrade fails, it returns an error 400 response.
WebSocket handlers
The websocket key defines event handlers to manage WebSocket connections.
? Connection Open (open)
const server = Bun.serve({ port: 8080, // defaults to $BUN_PORT, $PORT, $NODE_PORT otherwise 3000 ... });
Triggered when a client establishes a WebSocket connection.
- ws.send(...): sends a welcome message to the client who requested the connection..
✉️ Receiving a Message (message)
fetch(req, server) { const url = new URL(req.url); if (url.pathname === "/") return new Response(Bun.file("./index.html")); if (url.pathname === "/surprise") return new Response("?"); if (url.pathname === "/chat") { if (server.upgrade(req)) { return; // do not return a Response } return new Response("Upgrade failed", { status: 400 }); } return new Response("404!"); }
Triggered when the server receives a message from the client.
- ws.send(...): echoes back the received message with a confirmation.
⏹️ Connection Close (close)
open(ws) { console.log("? A new Websocket Connection"); ws.send("? Welcome baby"); }
Triggered when a WebSocket connection is closed.
Parameters:
- code: reason code for closing the connection.
- message: additional details about the closure.
? Drain Event (drain)
message(ws, message) { console.log("✉️ A new Websocket Message is received: " + message); ws.send("✉️ I received a message from you: " + message); }
The drain event is triggered when the WebSocket is ready to accept more data after being temporarily overwhelmed.
Log the server launch
close(ws, code, message) { console.log("⏹️ A Websocket Connection is CLOSED"); }
Logs the server's URL to the console once it's running.
Recap about how it works
- HTTP Requests: handles standard HTTP requests (e.g., serving a file or responding with a status).
- WebSocket Upgrade: upgrades HTTP connections to WebSocket connections when clients connect to /chat.
- Real-Time Communication: handles persistent communication between the server and clients using WebSocket events (open, message, close, drain).
Running the server
Once you have your index.ts file, you can start the server via bun run:
drain(ws) { console.log("DRAIN EVENT"); }
The server is ready and up and running. Now, we can implement the client.
Next steps
Now we understand the structure of the script for handling the WebSocket, the next steps are:
- implementing the HTML for the WebSocket client;
- Implementing broadcasting logic to forward messages from one client to all connected clients.
The above is the detailed content of WebSocket with JavaScript and Bun. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










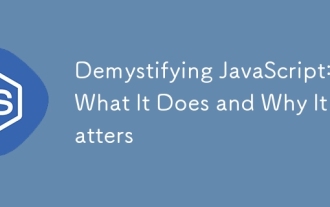
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
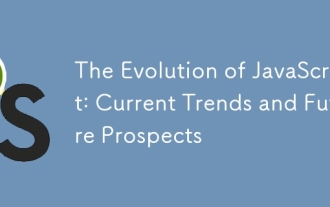
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
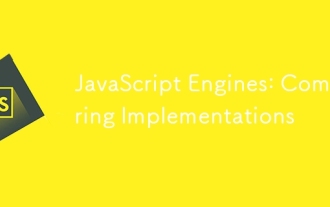
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
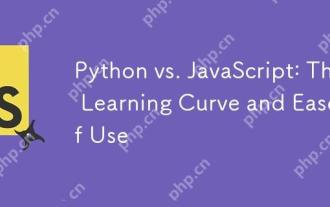
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
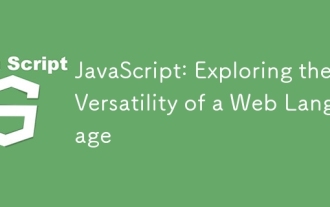
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
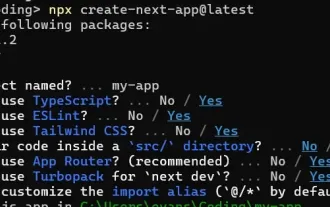
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
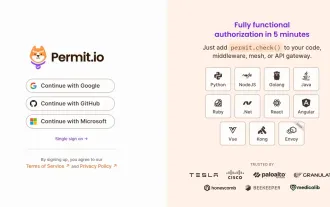
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
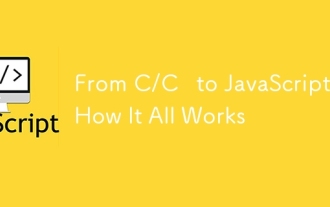
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
