


How Can I Overwrite Previous Output in Python's `print()` Function?
Overwriting Previous Output on Stdout
In Python, the default behavior of the print() function is to start a new line after each invocation. However, certain scenarios require overwriting the previous output on the same line.
Simple Overwrite
To overwrite the previous line, append 'r' (carriage return) to the end argument of print(). This return character moves the cursor to the beginning of the current line without starting a new one.
for x in range(10): print(x, end='\r') print()
Line Cleaning
When the new text is shorter than the previous line, it may leave remnants of the old text. To clear any remaining characters, append 'x1b[1K' (clear to end of line) to the end argument.
for x in range(75): print('*' * (75 - x), x, end='\x1b[1K\r') print()
Long Line Wrap
Line wrapping refers to the automatic continuation of a line past its end-of-line. To prevent line wrapping and force successive characters to overwrite existing ones, disable line wrapping using 'x1b[7l' and re-enable it using 'x1b[7h'.
print('\x1b[7l', end='') # disable line wrap print('\x1b[7h', end='') # re-enable line wrap
Note: Line wrap re-enabling must be done manually to prevent terminal breakage. Additionally, these solutions only control the length of the current line and do not overflow to subsequent lines.
The above is the detailed content of How Can I Overwrite Previous Output in Python's `print()` Function?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




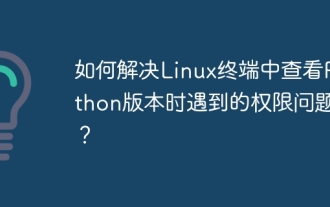
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
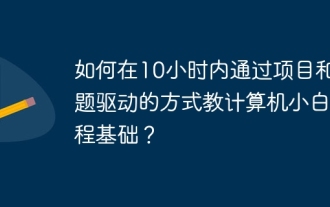
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
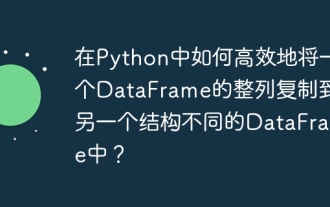
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
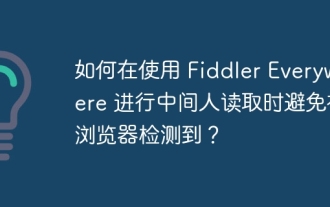
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
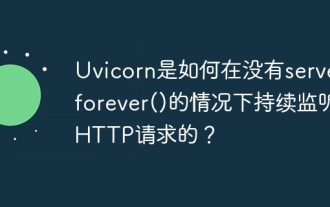
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
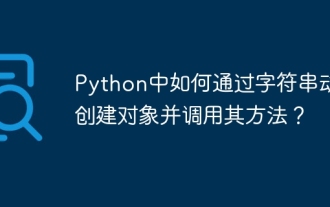
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
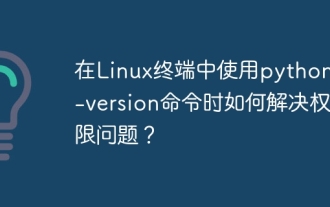
Using python in Linux terminal...
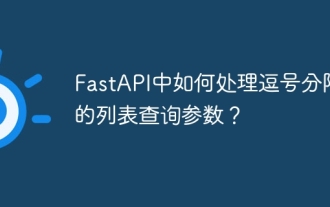
Fastapi ...
