


How Can JCSP Channels in Java Mimic Go Channels for Efficient Queuing?
Java Equivalent of Go Channels for Efficient Queuing Management
In Java, blocking queues are commonly used for concurrent data exchange. However, polling each queue individually for data availability can be inefficient. This article explores a solution that leverages the JCSP library to simulate the behavior of Go channels, enabling efficient and fair multiplexing of data.
JCSP's Alternative for Go's Select
JCSP provides an alternative mechanism for selecting data from multiple channels called Alternative. This construct allows a single consuming thread to switch between channels without continuously polling them. The fairSelect method of Alternative ensures that no channel is starved, guaranteeing fair access to data.
Example of Fair Multiplexing
The following Java code demonstrates a fair multiplexer that reads from multiple input channels and writes to a single output channel:
import org.jcsp.lang.*; public class FairPlex implements CSProcess { private final AltingChannelInput[] in; private final ChannelOutput out; public FairPlex(final AltingChannelInput[] in, final ChannelOutput out) { this.in = in; this.out = out; } public void run () { final Alternative alt = new Alternative (in); while (true) { final int index = alt.fairSelect (); out.write (in[index].read ()); } } }
Freedom from Deadlock
JCSP channels and Alternative have undergone formal analysis to guarantee freedom from deadlock. This ensures that Java programs using this library can be designed without the risk of deadlock.
Conclusion
By leveraging the JCSP library and its Alternative construct, Java developers can achieve the same efficient and fair multiplexing of data as Go channels. This approach allows for optimal utilization of threads and resources, resulting in more robust and scalable concurrent applications.
The above is the detailed content of How Can JCSP Channels in Java Mimic Go Channels for Efficient Queuing?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
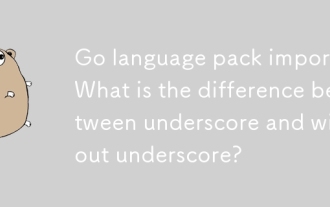
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
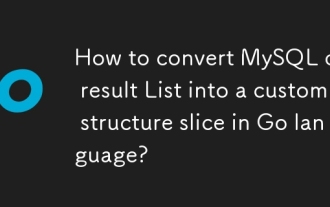
How to convert MySQL query result List into a custom structure slice in Go language?
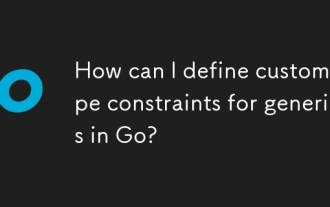
How can I define custom type constraints for generics in Go?
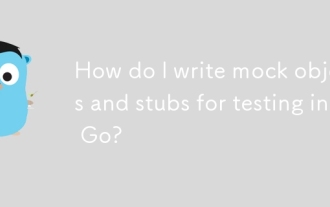
How do I write mock objects and stubs for testing in Go?
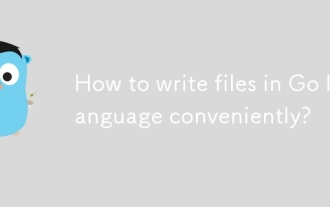
How to write files in Go language conveniently?
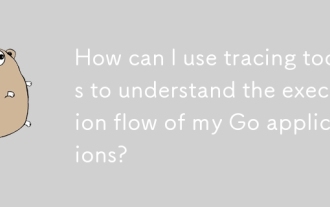
How can I use tracing tools to understand the execution flow of my Go applications?
