


How Can I Prevent Implicit Conversions in Non-Constructing Functions in C ?
Implicit Conversion Avoidance for Non-Constructing Functions
Consider a non-constructing function that expects an integer parameter but allows implicit casting from other types such as characters, booleans, and longs. To prevent this unintended behavior and ensure that the function only accepts parameters of the specified type, we can employ the following techniques:
C 11 and Later Method:
The most direct approach is to define a function template that matches all other types:
void function(int); // this will be selected for int only template <class T> void function(T) = delete; // C++11
This works because non-template functions with direct matching take precedence.
Pre-C 11 Technique:
Prior to C 11, an alternative solution involved creating a helper class to enforce the desired behavior:
// because this ugly code will give you compilation error for all other types class DeleteOverload { private: DeleteOverload(void*); }; template <class T> void function(T a, DeleteOverload = 0); void function(int a) {}
This technique relies on overloading multiple functions with similar signatures, where the function with the expected type takes precedence.
C 23 Update:
C 23 introduces a more concise and informative way to handle this situation:
void function(int) {} // this will be selected for int only template <class T> void function(T) { // since C++23 static_assert(false, "function shall be called for int only"); }
Using static_assert within a function template provides a clear error message when calling the function with an unexpected parameter type.
The above is the detailed content of How Can I Prevent Implicit Conversions in Non-Constructing Functions in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


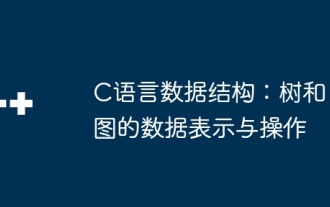
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
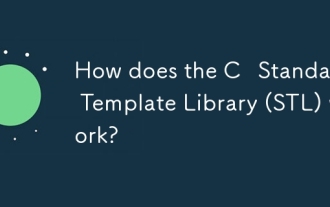
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
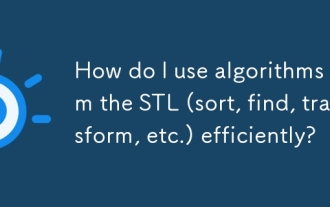
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
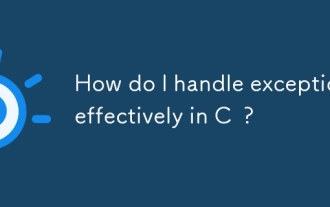
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
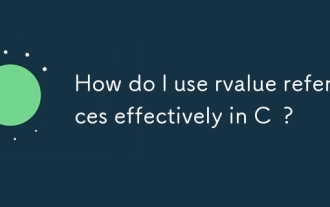
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
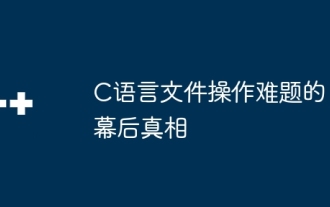
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
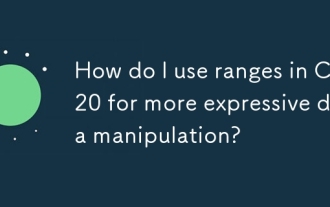
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
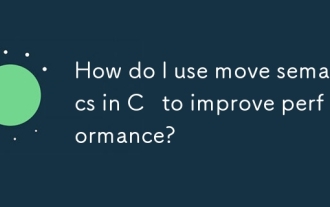
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
