


How Can I Group NumPy Array Elements Based on a Specific Column?
Group by Function in NumPy
NumPy provides several functions for array manipulation, including operations for grouping elements. One such operation is groupby, which allows you to group elements in an array based on a specified key.
Specific Problem
Consider the following array a:
a = array([[ 1, 275], [ 1, 441], [ 1, 494], [ 1, 593], [ 2, 679], [ 2, 533], [ 2, 686], [ 3, 559], [ 3, 219], [ 3, 455], [ 4, 605], [ 4, 468], [ 4, 692], [ 4, 613]])
Suppose you want to group the elements in a based on the first column. In this case, you would expect the output to be:
array([[[275, 441, 494, 593]], [[679, 533, 686]], [[559, 219, 455]], [[605, 468, 692, 613]]], dtype=object)
Solution
Although there is no direct groupby function in NumPy, it is possible to achieve this using the following approach:
# Sort the array by the first column a = a[a[:, 0].argsort()] # Find the unique values in the first column as keys keys = np.unique(a[:, 0]) # Create an array to hold the grouped elements grouped = [] # Iterate through the keys for key in keys: # Create a mask to select elements with the given key mask = (a[:, 0] == key) # Append the selected elements to the grouped array grouped.append(a[mask][:, 1])
This solution efficiently groups the elements in the a array based on the first column, even though it does not explicitly use a groupby function.
The above is the detailed content of How Can I Group NumPy Array Elements Based on a Specific Column?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










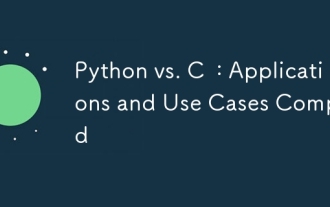
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
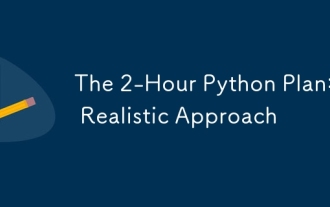
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
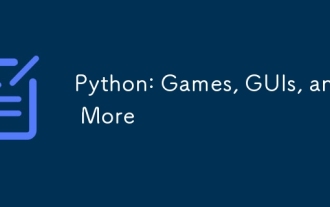
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
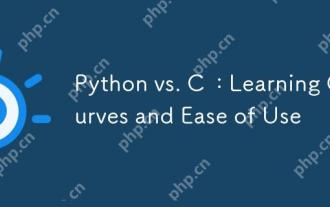
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
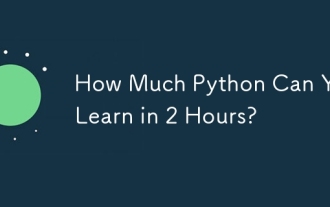
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
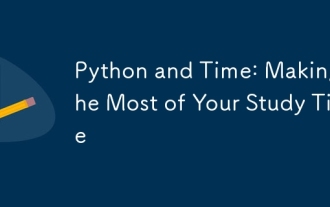
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
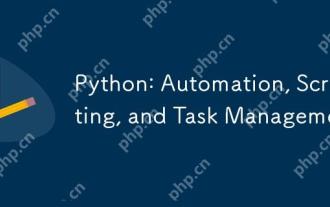
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
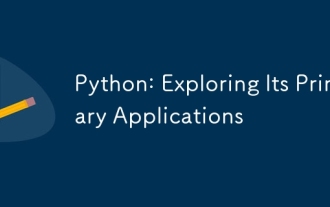
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
