How Can I Retrieve an Array of Regex Matches in Java?
Retrieval of Array of Regex Matches in Java
Problem:
When using regex expressions in Java, the primary method for checking matches only returns a boolean value, indicating whether a match is present or not. This can be limiting when attempting to capture and store multiple matching instances in an array.
Solution:
To construct an array of all string matches found within a given string using a regex expression, follow these steps:
- Create a Matcher: Utilize the Pattern and Matcher classes to create a Matcher object. This object allows you to iteratively find matches within the input string.
- Iterate and Collect Matches: Use the Matcher object's find() method to locate matches and the group() method to retrieve the matched substring. Continue this process until no more matches are found.
- Convert to Array: Once all matches have been collected, use the toArray() method to convert the list of matches into an array of strings. This array will contain all the strings that satisfied the specified regex expression.
Example Code:
import java.util.regex.Matcher; import java.util.regex.Pattern; import java.util.List; import java.util.ArrayList; public class RegexArray { public static void main(String[] args) { String input = "This is a sample string"; String regex = "[a-z]+"; // Create a Matcher object Matcher matcher = Pattern.compile(regex).matcher(input); // Collect matching substrings List<String> matches = new ArrayList<>(); while (matcher.find()) { matches.add(matcher.group()); } // Convert to string array String[] matchArray = matches.toArray(new String[0]); } }
By implementing these steps, you can effectively capture and store all instances that match a specified regex expression in an array, providing a structured and organized way to work with matched data.
The above is the detailed content of How Can I Retrieve an Array of Regex Matches in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










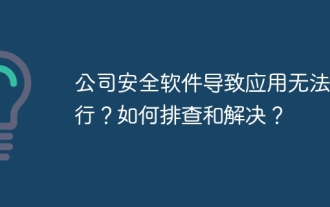
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
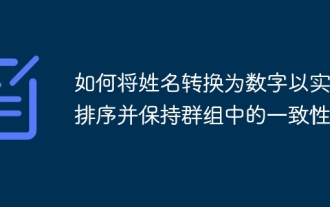
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
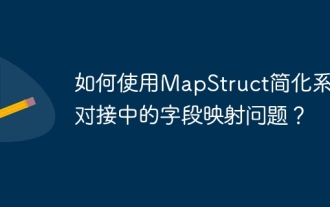
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
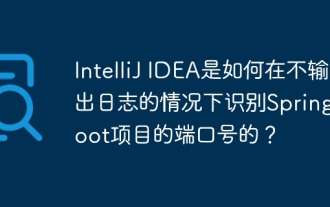
Start Spring using IntelliJIDEAUltimate version...
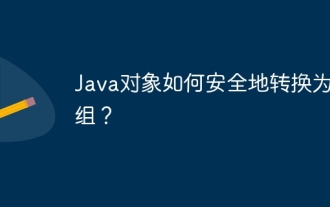
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
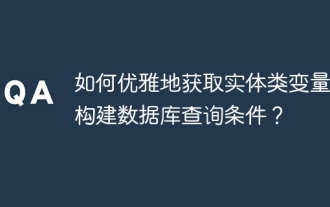
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
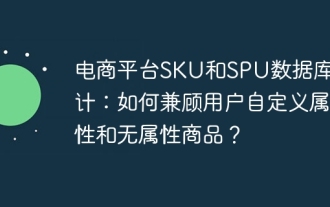
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
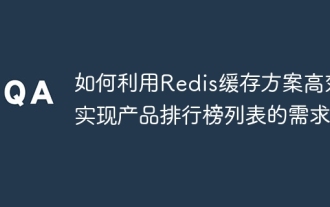
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
