How Can I Simulate C's 'static' Keyword for Local Variables in Go?
Preserving Local Variables in Go: An Alternative to C's 'static'
In Go, unlike C, there is no direct equivalent to the 'static' keyword for local variables. However, it is still possible to create local variables that retain their values between function calls by leveraging the concept of closures.
Closure to the Rescue
A closure in Go is a function that captures and shares the environment of the function in which it was created. This includes access to variables defined outside the closure's scope. By leveraging closures, we can simulate a similar behavior to that of a static local variable in C.
Example in Go
Consider the following code:
func main() { x := 0 func() { defer fmt.Println("x:", x) x++ }() for i := 0; i < 10; i++ { x++ } }
Here, we define a function (anonymous) that closes over the variable 'x' in the 'main' function. This captures the current value of 'x' when the function is executed.
Output:
x: 1 x: 2 x: 3 .... x: 10
As you can see, the value of 'x' increments within the closed-over function while remaining independent of the 'x' that is incremented in the 'main' function. This effectively behaves like a static local variable, preserving its value between function calls.
Note:
This approach works because the function captures the value of 'x' by-reference, rather than by-value. This ensures that any modifications made to 'x' within the function are reflected in the enclosing scope.
The above is the detailed content of How Can I Simulate C's 'static' Keyword for Local Variables in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










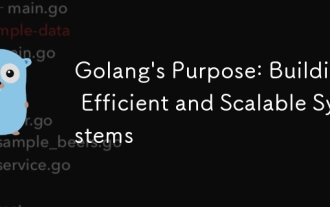
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
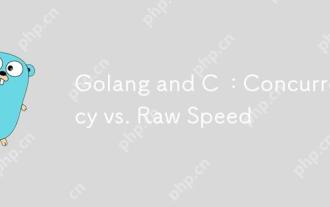
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
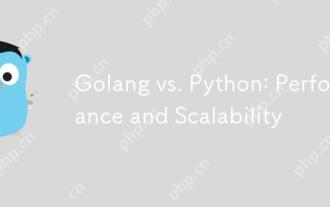
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
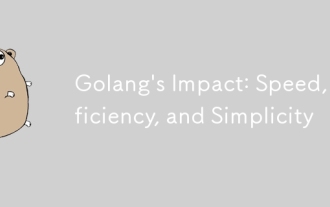
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
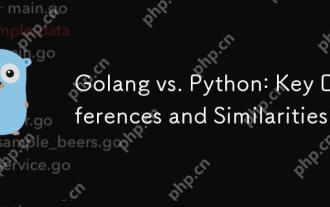
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
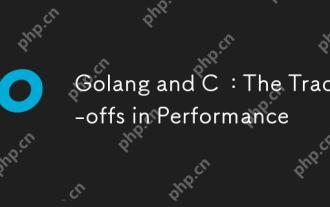
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
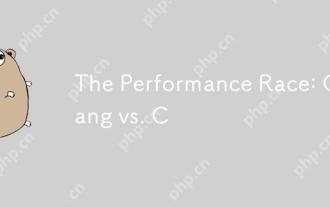
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
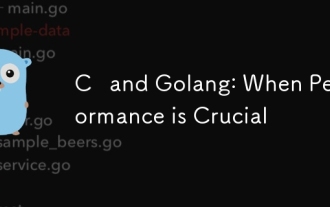
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
