


How Can I Catch Multiple Exceptions in a Single Python `except` Block?
Catching Multiple Exceptions in One Line with Python's Except Block
In Python, you can capture a variety of exceptions using the 'except' block. Traditionally, handling multiple exceptions involved using several individual 'except' clauses:
try: # Code that may fail except IDontLikeYouException: # Handle IDontLikeYouException except YouAreTooShortException: # Handle YouAreTooShortException
However, if you need to handle the same exception in response to multiple other exceptions, a more concise approach exists.
Catching Multiple Exceptions with a Tuple
By grouping exceptions into a tuple, you can handle their occurrences in a single 'except' block:
try: # Code that may fail except (IDontLikeYouException, YouAreBeingMeanException) as e: # Handle both exceptions
In this code, the tuple '(IDontLikeYouException, YouAreBeingMeanException)' specifies that the 'except' block will execute if either of those exceptions is raised.
Example Usage
Consider the code below:
def say_please(): print("Please...") try: raise IDontLikeYouException() except (IDontLikeYouException, YouAreBeingMeanException): say_please()
When the 'say_please()' function is called in the 'except' block, it prints "Please...". This demonstrates how handling multiple exceptions using a tuple simplifies exception handling.
Note for Python 2
In Python 2, you can optionally include a variable name after the closing parenthesis of the tuple, but it is not required and deprecated in Python 3. Instead, use 'as' to bind the exception object to a variable:
try: # Code that may fail except (IDontLikeYouException, YouAreBeingMeanException) as e: # Handle both exceptions
The above is the detailed content of How Can I Catch Multiple Exceptions in a Single Python `except` Block?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










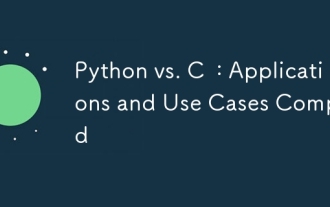
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
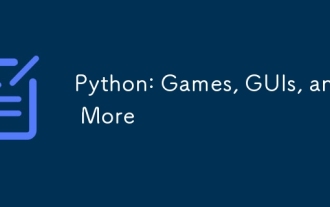
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
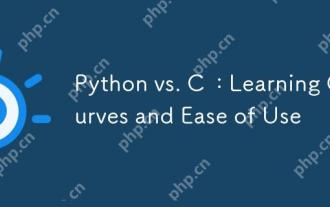
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
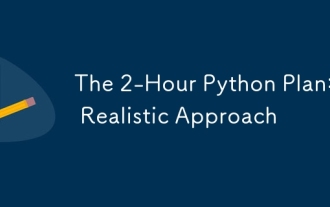
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
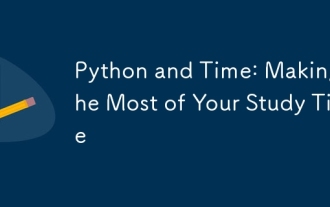
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
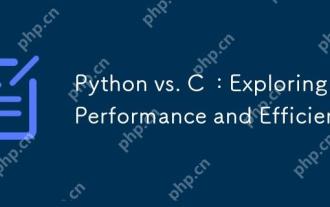
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
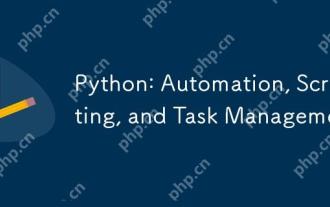
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
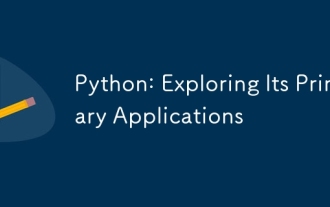
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
