


How Can I Accurately Calculate the Distance Between Two Coordinates in PHP?
Measuring the Distance Between Two Coordinates in PHP with Precision
Calculating the distance between two points on the Earth's surface requires precision, especially when astronomical distances are involved. The Haversine formula is a popular method for such calculations, but its implementation in the provided code seems to yield inaccurate results.
Haversine Formula Considerations
The Haversine formula employs spherical trigonometry to estimate the distance between two geographical coordinates. While it provides accurate approximations for short distances, it becomes less reliable for extreme distances (e.g., near the antipodes).
Alternative Calculation Formula: Vincenty
To overcome the limitations of the Haversine formula, especially for large-scale distance calculations, the Vincenty formula offers improved accuracy. It uses iterative methods to compute the geodesic distance along the ellipsoid that approximates the Earth's surface.
Implementation
Below is an implementation of the Vincenty formula in PHP:
function vincentyGreatCircleDistance($latFrom, $lonFrom, $latTo, $lonTo, $earthRadius = 6371000) { // Convert to radians $latFrom = deg2rad($latFrom); $lonFrom = deg2rad($lonFrom); $latTo = deg2rad($latTo); $lonTo = deg2rad($lonTo); $lonDelta = $lonTo - $lonFrom; $a = pow(cos($latTo) * sin($lonDelta), 2) + pow(cos($latFrom) * sin($latTo) - sin($latFrom) * cos($latTo) * cos($lonDelta), 2); $b = sin($latFrom) * sin($latTo) + cos($latFrom) * cos($latTo) * cos($lonDelta); $angle = atan2(sqrt($a), $b); return $angle * $earthRadius; }
This implementation outputs the distance in meters, consistent with the input $earthRadius. For miles, you can pass in 3959 miles as the $earthRadius parameter. Utilizing the Vincenty formula ensures reliable distance calculations, even for extreme distances.
The above is the detailed content of How Can I Accurately Calculate the Distance Between Two Coordinates in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
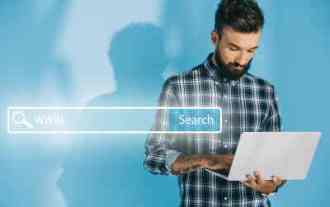
11 Best PHP URL Shortener Scripts (Free and Premium)
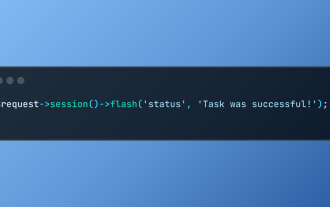
Working with Flash Session Data in Laravel
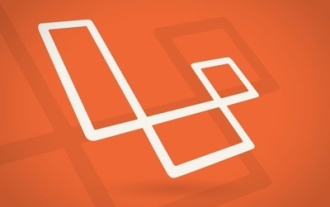
Build a React App With a Laravel Back End: Part 2, React
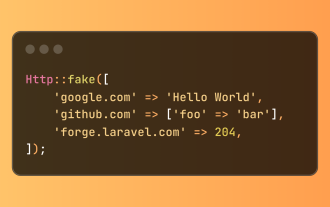
Simplified HTTP Response Mocking in Laravel Tests
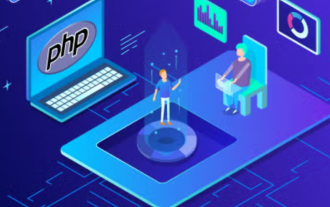
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
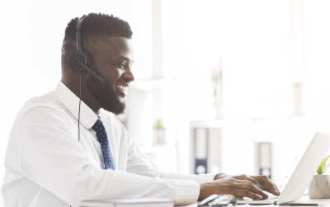
12 Best PHP Chat Scripts on CodeCanyon
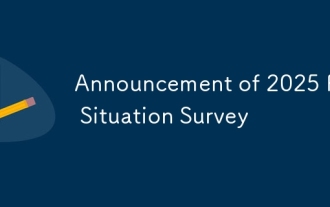
Announcement of 2025 PHP Situation Survey
