How Can I Efficiently Sort HashMap Values in Java?
Sorting HashMaps in Java: A Comprehensive Guide
Sorting a HashMap can be a useful technique for organizing and retrieving data efficiently. However, it's important to understand the limitations and considerations involved in this process.
Sorting HashMaps by Values
If you wish to sort a HashMap based on the values within the ArrayLists, you will need to implement custom sorting logic. Here's an example:
Map<String, Person> people = new HashMap<>(); Person jim = new Person("Jim", 25); Person scott = new Person("Scott", 28); Person anna = new Person("Anna", 23); people.put(jim.getName(), jim); people.put(scott.getName(), scott); people.put(anna.getName(), anna); // Convert HashMap values to a list for sorting List<Person> peopleByAge = new ArrayList<>(people.values()); // Sort the list using the Person::getAge comparator Collections.sort(peopleByAge, Comparator.comparing(Person::getAge)); // Iterate over the sorted list for (Person p : peopleByAge) { System.out.println(p.getName() + "\t" + p.getAge()); }
Alternatives to Sorting HashMaps
It's worth noting that sorting HashMaps can be computationally expensive, especially for large datasets. Consider the following alternatives:
- Use a TreeMap: If you only need to maintain keys in sorted order, use a TreeMap instead of a HashMap.
- Filter and Sort Values: If you want to sort values within a HashMap, filter the values by applying a predicate and then sort the resulting list.
- Insert Values into a Sorted Collection: Insert elements into a HashMap that maintains a sorted order, such as a TreeSet. However, the semantics of sets and lists differ.
Conclusion
Sorting HashMaps in Java requires careful consideration. Custom sorting logic can be implemented to sort values within ArrayLists, but this may be inefficient for large datasets. Explore alternatives such as TreeMaps, filtering, or using sorted collections for optimal performance.
The above is the detailed content of How Can I Efficiently Sort HashMap Values in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










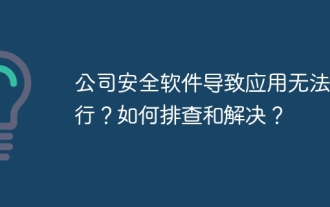
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
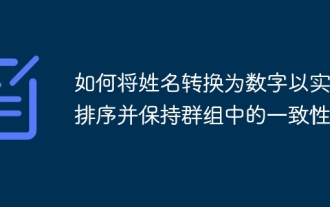
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
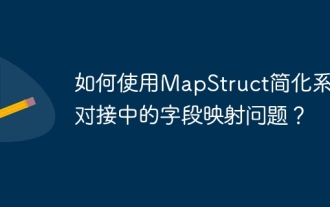
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
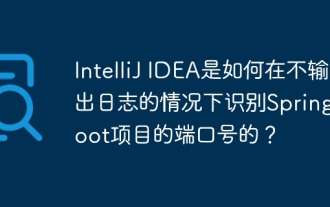
Start Spring using IntelliJIDEAUltimate version...
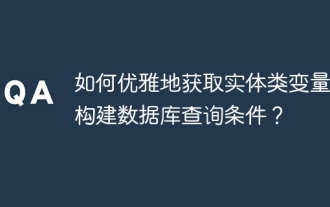
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
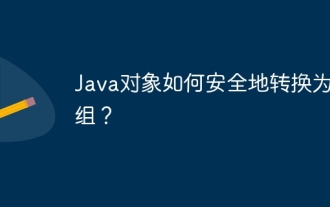
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
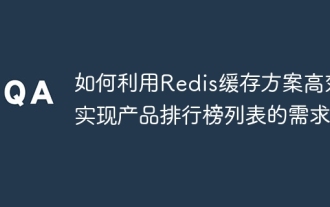
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
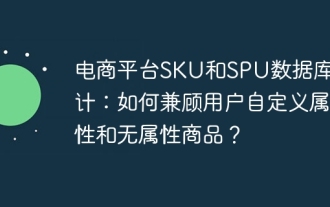
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
