How Can I Efficiently Add Leading Zeroes to Dates in JavaScript?
Dec 03, 2024 pm 10:08 PMAdding Leading Zeroes to Dates in Javascript
When working with dates, it's often necessary to represent them in a specific format, including leading zeroes. This can be achieved by modifying the following script, which calculates the date for 10 days in advance in the format dd/mm/yyyy:
var MyDate = new Date(); var MyDateString = new Date(); MyDate.setDate(MyDate.getDate() + 10); MyDateString = MyDate.getDate() + '/' + (MyDate.getMonth() + 1) + '/' + MyDate.getFullYear();
To add leading zeroes to the day and month components, we can insert the following rules:
if (MyDate.getMonth() < 10) getMonth = '0' + getMonth;
if (MyDate.getDate() < 10) get.Date = '0' + getDate;
However, this approach is not efficient and can be simplified. Instead, we can use the slice(-2) method to get the last two characters of the string:
var MyDate = new Date(); var MyDateString; MyDate.setDate(MyDate.getDate() + 20); MyDateString = ('0' + MyDate.getDate()).slice(-2) + '/' + ('0' + (MyDate.getMonth()+1)).slice(-2) + '/' + MyDate.getFullYear();
This method provides a cleaner and more efficient way to add leading zeroes to the date components, ensuring the desired format is maintained.
The above is the detailed content of How Can I Efficiently Add Leading Zeroes to Dates in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
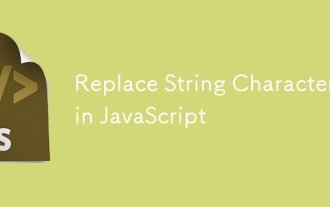
Replace String Characters in JavaScript
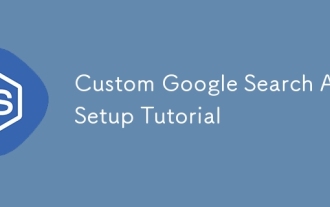
Custom Google Search API Setup Tutorial
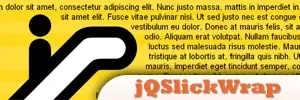
8 Stunning jQuery Page Layout Plugins
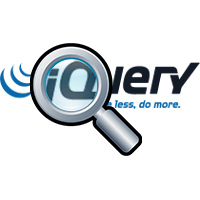
Improve Your jQuery Knowledge with the Source Viewer
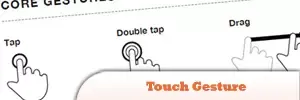
10 Mobile Cheat Sheets for Mobile Development
