How Can I Efficiently Extract a Filename from a File Path in Go?
Dec 03, 2024 pm 10:12 PMExtracting Filename from Path
In Go, the file name and path are often stored together in a string. Removing the path to obtain just the file name can be a common task. This article addresses such a scenario, explaining how to accomplish it effectively.
The initial approach of using strings.LastIndex to identify the last slash character is not ideal because it returns the character's index instead of the desired file name. To correctly isolate the file name, we recommend utilizing the filepath.Base function.
Using filepath.Base for Filename Extraction
The filepath.Base function accepts a path and extracts the final element, which typically represents the file name. It is an efficient method for this specific task.
import ( "fmt" "os" "path/filepath" ) func main() { path := "/some/path/to/remove/file.name" file := filepath.Base(path) fmt.Println(file) // Output: file.name }
Playground for Verification
We provide a Golang playground for you to experiment with this code: http://play.golang.org/p/DzlCV-HC-r.
By employing filepath.Base, you can easily separate the filename from its path in Go, adhering to the convention of representing file names without the preceding directory path.
The above is the detailed content of How Can I Efficiently Extract a Filename from a File Path in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
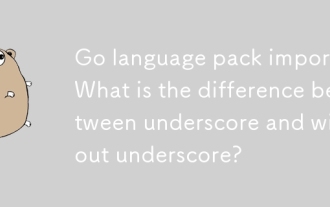
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
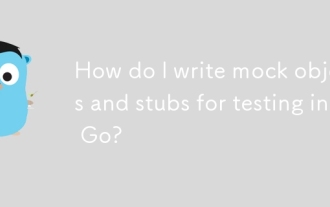
How do I write mock objects and stubs for testing in Go?
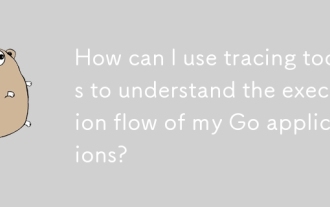
How can I use tracing tools to understand the execution flow of my Go applications?
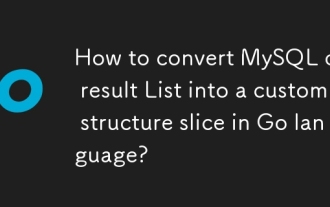
How to convert MySQL query result List into a custom structure slice in Go language?
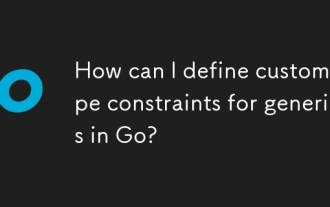
How can I define custom type constraints for generics in Go?
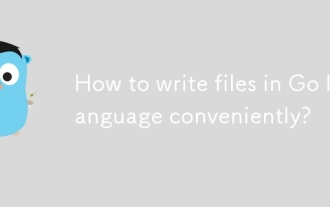
How to write files in Go language conveniently?
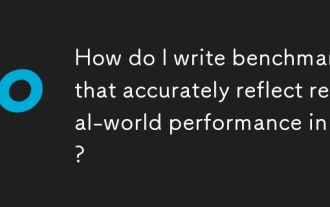
How do I write benchmarks that accurately reflect real-world performance in Go?
