


Why Does Assigning to a Map in Go Result in a 'runtime error: assignment to entry in nil map' and How Can It Be Fixed?
Runtime Error in Map Assignment
Question:
While attempting to convert a map to a YAML file, an error is encountered stating "runtime error: assignment to entry in nil map." Review the following code snippet:
package main import ( "fmt" "gopkg.in/yaml.v2" ) type T struct { cn string street string } func main() { names := []string{"kasi", "remya", "nandan"} m := make(map[string]map[string]T, len(names)) for _, name := range names { //t := T{cn: "Chaithra", street: "fkmp"} m["uid"][name] = T{cn: "Chaithra", street: "fkmp"} } fmt.Println(m) y, _ := yaml.Marshal(&m) fmt.Println(string(y)) //fmt.Println(m, names) }
What is causing this error and how can it be resolved?
Answer:
The issue lies in the initialization of the inner map within the outer map. In the example provided, the map m["uid"] is not initialized, resulting in a nil map when attempting to assign the name to it.
To initialize the inner map, add the following line before the for loop:
m["uid"] = make(map[string]T)
This creates the inner map and allows the name to be assigned to it without encountering a nil map error.
The corrected code becomes:
func main() { names := []string{"kasi", "remya", "nandan"} m := make(map[string]map[string]T, len(names)) m["uid"] = make(map[string]T) // Initialize the inner map for _, name := range names { m["uid"][name] = T{cn: "Chaithra", street: "fkmp"} } fmt.Println(m) y, _ := yaml.Marshal(&m) fmt.Println(string(y)) //fmt.Println(m, names) }
The above is the detailed content of Why Does Assigning to a Map in Go Result in a 'runtime error: assignment to entry in nil map' and How Can It Be Fixed?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










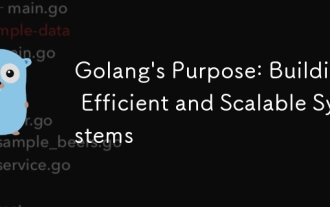
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
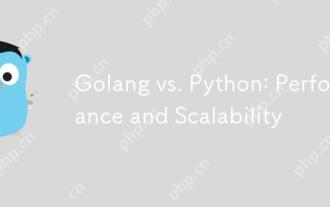
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
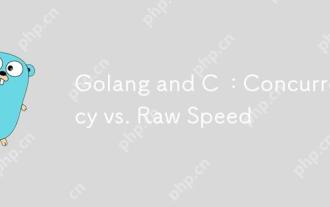
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
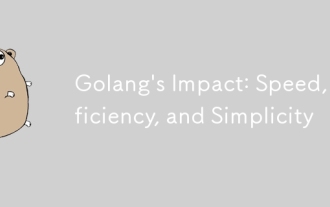
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
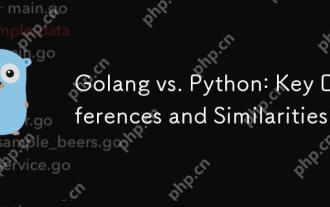
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
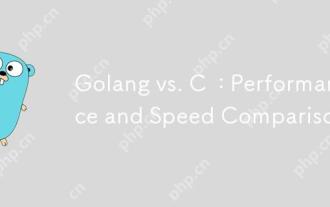
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
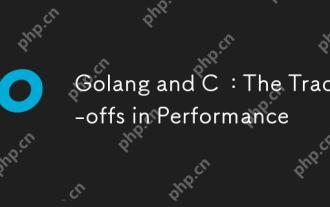
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
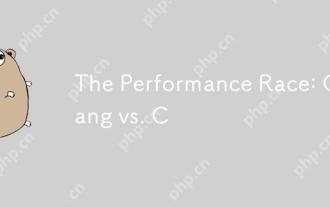
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
