


How Can I Mock or Abstract the Filesystem in Go for Testing and Custom File Handling?
Mocking/Abstracting the Filesystem in Go
In Go, it is possible to mock or abstract the filesystem to facilitate testing and implement custom file handling behaviors. Specifically, you can log each read/write operation performed by your Go application on the underlying OS, and even replace the filesystem entirely with one that resides in memory.
To achieve this, follow these steps:
1. Define a FileSystem Interface:
Start by defining an interface named fileSystem that abstracts the methods required for working with files, including Open and Stat.
type fileSystem interface { Open(name string) (file, error) Stat(name string) (os.FileInfo, error) }
2. Implement the Interface for the OS Filesystem:
Create a type for the OS filesystem called osFS that implements the fileSystem interface using the os package.
type osFS struct{} func (osFS) Open(name string) (file, error) { return os.Open(name) } func (osFS) Stat(name string) (os.FileInfo, error) { return os.Stat(name) }
3. Define a File Interface:
Introduce a file interface to abstract the operations that can be performed on a file. This includes methods like io.Closer, io.Reader, and io.Seeker.
type file interface { io.Closer io.Reader io.ReaderAt io.Seeker Stat() (os.FileInfo, error) }
4. Set the Default FileSystem:
Declare a global fileSystem variable and initialize it with osFS as the default implementation.
var fs fileSystem = osFS{}
5. Incorporate the FileSystem Abstraction into Your Code:
Modify your code to accept a fileSystem argument in its functions, enabling you to use either the default OS filesystem or a custom one.
func myFunction(name string, fs fileSystem) { f, err := fs.Open(name) // ... }
By following these steps, you can seamlessly mock or abstract the filesystem in Go, providing greater control and flexibility for testing and custom file system implementations.
The above is the detailed content of How Can I Mock or Abstract the Filesystem in Go for Testing and Custom File Handling?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










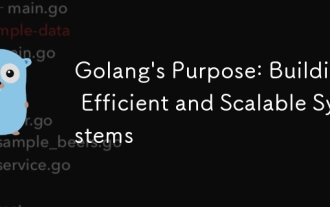
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
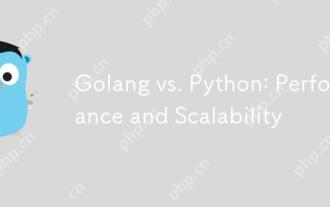
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
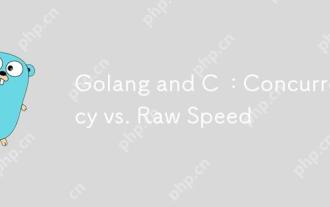
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
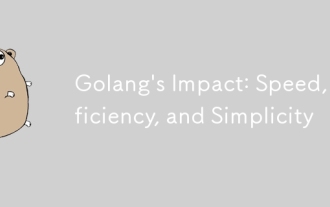
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
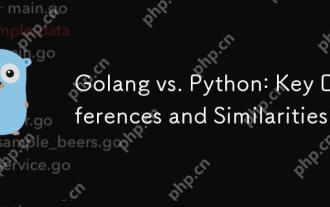
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
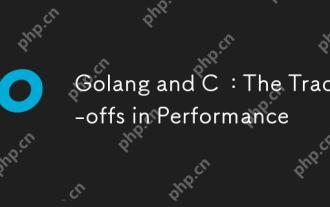
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
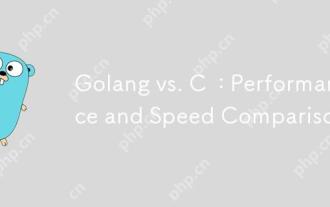
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
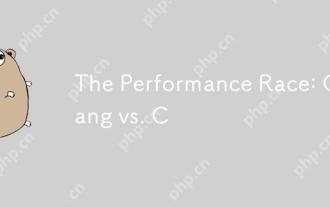
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
