Python Day - Looping-Examples and Tasks
Task:1
#Print Numbers #Write a program to print numbers from 1 to 10 using a while loop. def print_numbers(num): no=1 while no<=num: print(no,end=' ') no+=1 print_numbers(10)
Output:
1 2 3 4 5 6 7 8 9 10
Task:2
#Sum of N Numbers #Write a program to calculate the sum of the first NN natural numbers using a while loop. def nat_num(N): sum = 0 natural_no = 1 while natural_no <= N: sum += natural_no natural_no += 1 return sum N = int(input("Enter the no: ")) print(nat_num(N))
Output:
Enter the no: 10 Sum of 10 natural numbers are 55
Task:3
#Find even numbers between 1 to 50 def even_number(num): no=2 while no<=num: print(no, end=" ") no+=2 num=int(input("Enter the number: ")) even_number(num)
Output:
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50
Task:4
#Find odd numbers between 1 and NN def odd_number(num): no=1 while no<=num: print(no, end=" ") no+=2 num=int(input("Enter the number:")) odd_number(num)
Output:
Enter the number:15 1 3 5 7 9 11 13 15
Task:5
#Multiplication table of number NN #NN-2 no=1 while no<=10: print(f'{no}*2={no*2}') no+=1
Output:
1*2=2 2*2=4 3*2=6 4*2=8 5*2=10 6*2=12 7*2=14 8*2=16 9*2=18 10*2=20
Factorial:
Write a program to calculate the factorial of a given number using a while loop.
Case-1
no = 1 factorial = 1 factorial = factorial * no #1 no = no+1 #2 factorial = factorial * no #2 no = no+1 #3 factorial = factorial * no #6 no = no+1 #4 factorial = factorial * no #24 no = no+1 #5 factorial = factorial * no #120 print(factorial)
Case-2
def find_factorial(num): no=1 factorial=1 while no<=num: factorial=factorial*no no+=1 return factorial num=int(input("Enter the number:")) print(find_factorial(num))
For scenarios output will be same
Output:
Enter the number:5 120
Sum of digits:
Find the sum of the digits of a given number (e.g., 123 → 1 2 3=61 2 3=6).
def sum_of_digits(num): sum=0 while num>0: sum=sum+num%10 num=num//10 return sum num=int(input("Enter the number:")) print(sum_of_digits(num))
Output:
Enter the number:123 6
Count Digits
To count the number of digits in a given number (e.g., 12345 → 5 digits).
def count_of_digits(no): count=0 while no>0: no=no//10 count+=1 return count no=int(input("Enter the number:")) print(count_of_digits(no))
Output:
Enter the number:12345 5
Reverse numbers
To reverse a number
def reverse_no(num): reverse = 0 while num>0: reverse = (reverse*10) + num%10 num = num//10 return reverse print(reverse_no(1234))
Output:
Enter the number:12345 54321
Check Palindrome
check if a given number is a palindrome (e.g., 121 → palindrome, 123 → not a palindrome).
def palindrome(num): count=0 while num>0: count=count*10+num%10 num=num//10 return count num=int(input("Enter the number:")) result=palindrome(num) if result==num: print("Palindrome") else: print("Not palindrome")
Output:
Enter the number:121 Palindrome Enter the number:123 Not palindrome
Find power
def find_power(base,power): result=1 while power>=1: result=result*base power-=1 return result base=int(input("Enter the base number:")) power=int(input("Enter the power number:")) result=find_power(base,power) print(result)
Output:
Enter the base number:5 Enter the power number:2 25
Armstrong Number
Example:
def count_of_digits(num): count=0 while num>0: num=num//10 count+=1 return count def find_power(base,power): result=1 while power>=1: result=result*base power-=1 return result def find_armstrong(num): armstrong=0 while num>0: rem=num%10 result= find_power(rem,count) armstrong=armstrong+result num=num//10 return armstrong num=int(input("Enter the number:")) count=count_of_digits(num) armstrong_result=find_armstrong(num) if armstrong_result==num: print("Armstrong") else: print("Not armstrong")
Output:
Enter the number:135 Not armstrong Enter the number:153 Armstrong
The above is the detailed content of Python Day - Looping-Examples and Tasks. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










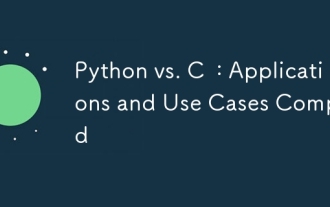
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
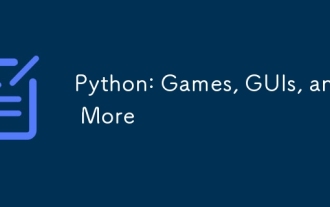
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
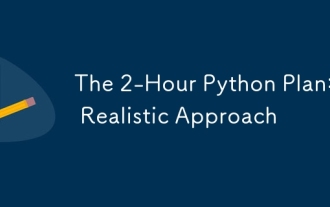
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
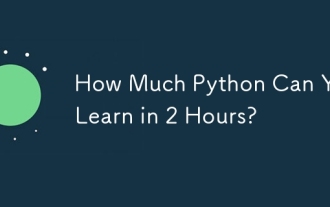
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
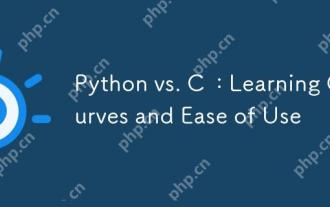
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
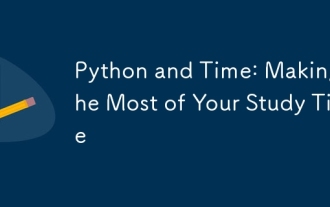
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
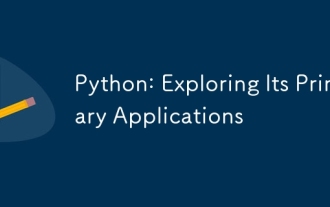
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
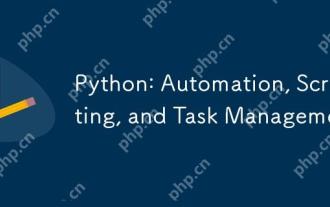
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
