


How Can Python's `os.path` Module Efficiently Extract Filenames from Various Paths?
Extract File Names from Paths: A Cross-Platform Solution
Extracting file names from paths can be a challenge, especially when dealing with different operating systems and path formats. Fortunately, Python offers a robust and versatile library for path manipulation: the os.path module.
The os.path.basename() function is specifically designed to extract file names from paths. It accepts a path as an argument and returns the file name as a string. The path can be absolute or relative, and can use any valid path format for the operating system.
Here are several examples of how os.path.basename() can be used to extract file names from various path formats:
>>> from os.path import basename # Absolute paths print(basename("/a/b/c/")) print(basename("C:\a\b\c\")) # Relative paths print(basename("a/b/c")) print(basename("..\a\b\c")) # Paths with trailing slashes print(basename("/a/b/c/")) print(basename("C:\a\b\c\")) # Paths with .. references print(basename("a/b/../../a/b/c/")) print(basename("a/b/../../a/b/c")) # Output: # c # c # c # c # c # c # c # c
Note: When using os.path.basename() on a POSIX system to get the base name from a Windows-styled path (e.g. "C:\my\file.txt"), the entire path will be returned. This is due to the fact that Windows paths are not standardized in the same way as POSIX paths.
The above is the detailed content of How Can Python's `os.path` Module Efficiently Extract Filenames from Various Paths?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




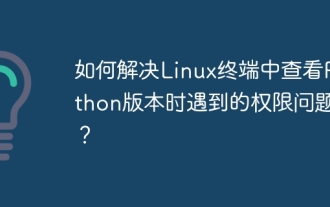
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
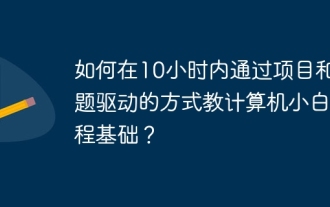
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
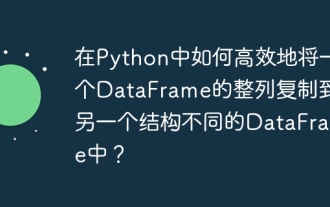
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
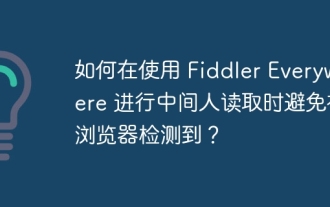
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
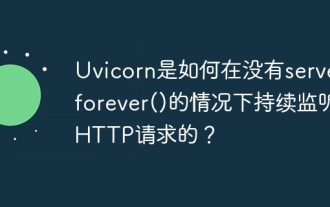
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
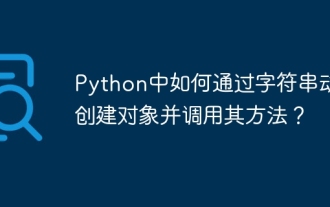
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
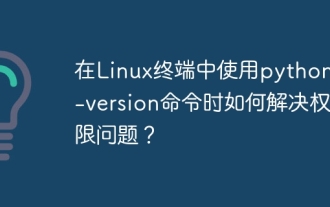
Using python in Linux terminal...
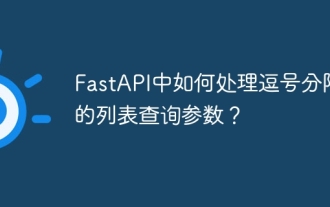
Fastapi ...
