


What are Python\'s Named Tuples and How Do They Compare to Regular Tuples?
What are Named Tuples in Python?
Named tuples are lightweight and easy-to-create object types that enhance the usability of tuples by providing named attributes. Let's delve into their usage and comparison with regular tuples.
Creation and Usage of Named Tuples
To create named tuples, we use the collections.namedtuple factory function. For instance, to define a named tuple for points:
from collections import namedtuple Point = namedtuple('Point', 'x y')
Instances of this named tuple can be created like regular tuples:
pt1 = Point(1.0, 5.0) pt2 = Point(2.5, 1.5)
The benefits of using named tuples become evident when referencing their attributes:
line_length = sqrt((pt1.x - pt2.x)**2 + (pt1.y - pt2.y)**2) # Object-like syntax
Advantages of Named Tuples vs. Regular Tuples
- Improved Readability: Named tuple attributes provide explicit names, making code easier to understand.
- Object-Like Notation: Named tuple instances can be accessed via object-like variable dereferencing (e.g., pt1.x), eliminating the need for index referencing.
- Type Checking: Named tuples allow for type checking, reducing the risk of errors due to index mismatches.
Use Cases for Named Tuples
Named tuples are recommended when:
- Data structures consist of immutable value types.
- Object notation and named attributes enhance readability.
- Simple value types are passed as parameters to functions, improving function clarity.
Beyond Basic Named Tuples
Named tuples can even replace immutable classes with only fields. They can also serve as base classes for custom named tuples:
class Point(namedtuple('Point', 'x y')): [...] # Define additional methods
Named Lists and Mutable Named Tuples
There is no built-in equivalent for "named lists" in Python. However, for mutable record types, there exist recipes or third-party modules that allow setting new values to attributes:
from rcdtype import recordtype Point = recordtype('Point', 'x y') pt1 = Point(1.0, 5.0) pt1.x = 2.0 # Mutable!
Named tuples can be manipulated like dictionaries using pt1._asdict(), providing easy access to their fields and compatibility with dictionary operations.
Conclusion
Named tuples are a powerful tool in Python, providing a clean and intuitive way to represent data, while offering improved readability, type checking, and customizability. Whether you are working with simple value types or complex record structures, named tuples can enhance the efficiency and clarity of your code.
The above is the detailed content of What are Python\'s Named Tuples and How Do They Compare to Regular Tuples?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










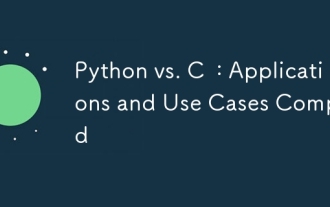
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
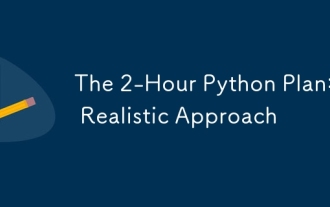
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
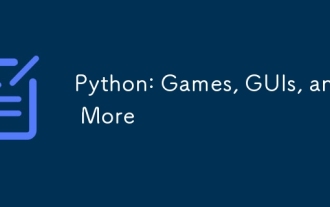
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
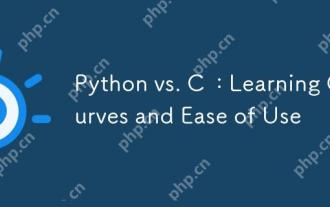
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
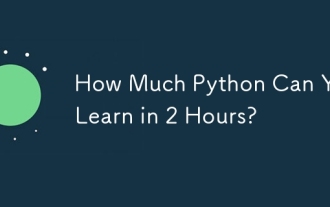
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
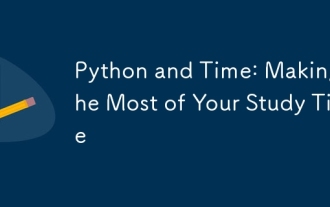
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
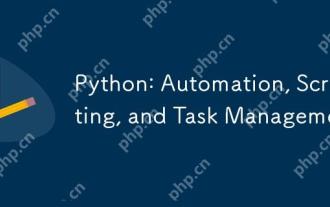
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
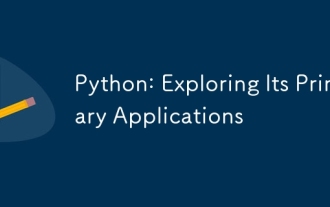
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
