


Why Does My String Search in Python Text Files Always Return True?
Search for a String within Text Files
In an attempt to determine the presence of a specific string within a text file, a program may encounter unexpected behavior. To rectify this issue, it's crucial to understand the underlying reason behind the incorrect results.
Original Code:
def check(): datafile = file('example.txt') found = False for line in datafile: if blabla in line: found = True break check() if True: print "true" else: print "false"
Reason for False Results:
The provided code consistently returns True regardless of the string's presence in the file because the evaluation of 'if True' in the following block is not tied to the logic of the preceding loop.
if True: print "true" else: print "false"
Python with Open:
An alternative approach to reading a text file is to utilize the 'with' statement in conjunction with the 'open()' function. This method creates a 'file-like' object that automatically handles file closing.
with open('example.txt') as f: if 'blabla' in f.read(): print("true")
Using Memory Mapping:
Another technique for working with text files is memory mapping. This approach reads the entire file into memory as a string-like object, enabling faster access and the possibility of using regular expressions.
import mmap with open('example.txt', 'rb', 0) as file, \ mmap.mmap(file.fileno(), 0, access=mmap.ACCESS_READ) as s: if s.find(b'blabla') != -1: print('true')
By following these suggestions, you can accurately search for strings within text files and obtain the expected results.
The above is the detailed content of Why Does My String Search in Python Text Files Always Return True?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










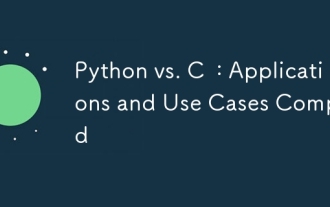
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
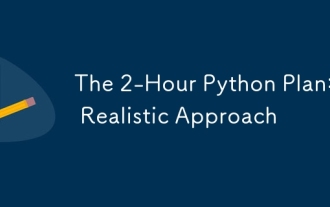
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
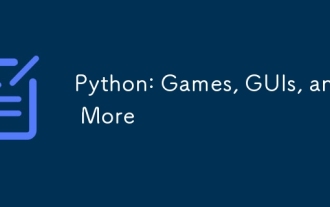
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
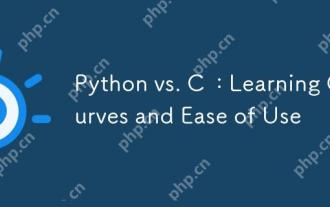
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
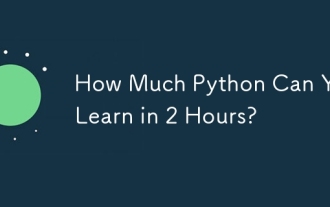
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
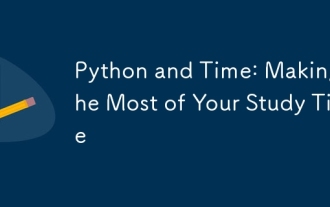
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
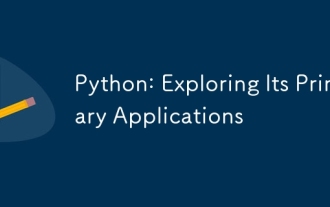
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
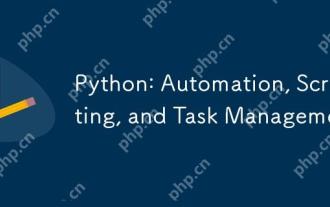
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
