How Do I Convert Strings and Byte Arrays Using Different Encodings in Java?
Converting Strings and Byte Arrays with Encodings
In Java, strings can be encoded into byte arrays, and byte arrays can be decoded into strings using different encodings.
Encoding a String into a Byte Array
To encode a string into a byte array using UTF-8 encoding:
String s = "my string"; byte[] b = s.getBytes(StandardCharsets.UTF_8);
Other commonly used encodings include US-ASCII:
byte[] b = s.getBytes(StandardCharsets.US_ASCII);
Decoding a Byte Array into a String
To decode a byte array into a string using UTF-8 decoding:
byte[] b = {(byte) 99, (byte) 97, (byte) 116}; String s = new String(b, StandardCharsets.UTF_8);
Similarly, for US-ASCII decoding:
String s = new String(b, StandardCharsets.US_ASCII);
Remember to use the appropriate encoding name for your specific use case. By leveraging these encoding methods, you can seamlessly convert between string and byte array representations with different character encodings.
The above is the detailed content of How Do I Convert Strings and Byte Arrays Using Different Encodings in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










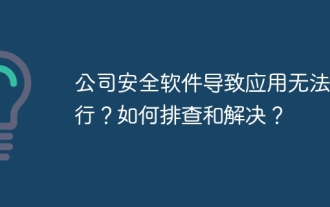
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
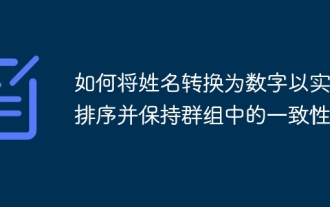
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
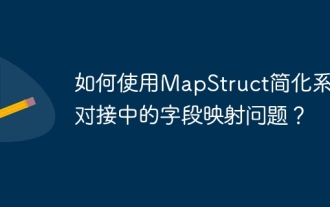
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
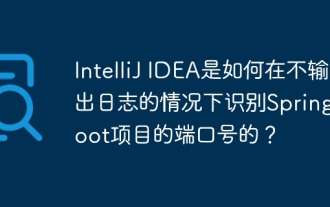
Start Spring using IntelliJIDEAUltimate version...
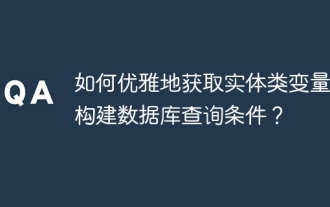
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
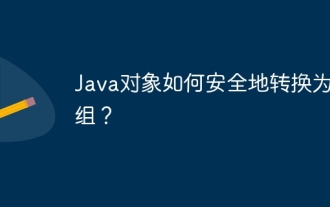
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
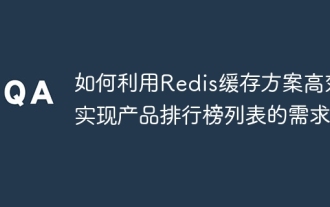
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
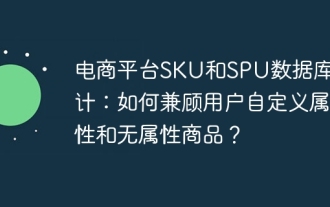
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
